In this tutorial, we will create a navbar menu in React using Ant Design 5. We will demonstrate how to implement an Ant Design 5 navbar with icons and provide an example of a responsive navbar using React and Ant Design 5.
install & setup vite + react + typescript + ant design 5
React Ant Design 5 Navbar Example
1. Create react ant design 5 simple navbar using react-antd Layout, Menu, Button component.
import React from 'react';
import { Layout, Menu, Button } from 'antd';
const { Header } = Layout;
const NavBar = () => (
<Layout className="layout">
<Header style={{ display: 'flex', justifyContent: 'space-between' }}>
<Menu theme="dark" mode="horizontal" defaultSelectedKeys={['1']}>
<Menu.Item key="1" >Home</Menu.Item>
<Menu.Item key="2" >Profile</Menu.Item>
<Menu.Item key="3">Settings</Menu.Item>
</Menu>
<div>
<Button type="primary" style={{ marginRight: '10px' }}>Sign in</Button>
<Button>Sign up</Button>
</div>
</Header>
</Layout>
);
export default NavBar;

2. react ant design 5 navbar with icons.
import React from 'react';
import { Layout, Menu, Button } from 'antd';
import {
HomeOutlined,
UserOutlined,
SettingOutlined,
} from '@ant-design/icons';
const { Header } = Layout;
const NavBarExample = () => (
<Layout className="layout">
<Header style={{ display: 'flex', justifyContent: 'space-between' }}>
<div className="logo" style={{ color: 'white' }}> Logo</div>
<Menu theme="dark" mode="horizontal" defaultSelectedKeys={['1']}>
<Menu.Item key="1" icon={<HomeOutlined />}>Home</Menu.Item>
<Menu.Item key="2" icon={<UserOutlined />}>Profile</Menu.Item>
<Menu.Item key="3" icon={<SettingOutlined />}>Settings</Menu.Item>
</Menu>
<div>
<Button type="primary" style={{ marginRight: '10px' }}>Sign in</Button>
<Button>Sign up</Button>
</div>
</Header>
</Layout>
);
export default NavBarExample;

3. react ant design 5 responsive navbar using react-antd Layout, Menu, Button, Drawer, Row, Col component.
import React, { useState } from "react";
import { Layout, Menu, Button, Drawer, Row, Col } from "antd";
import {
HomeOutlined,
UserOutlined,
SettingOutlined,
MenuOutlined,
} from "@ant-design/icons";
const { Header } = Layout;
const ResponsiveNav = () => {
const [visible, setVisible] = useState(false);
const showDrawer = () => {
setVisible(true);
};
const onClose = () => {
setVisible(false);
};
return (
<Layout className="layout">
<Header style={{ padding: 0 }}>
<Row justify="space-between" align="middle">
<Col xs={20} sm={20} md={4}>
<div
className="logo"
style={{ color: "white", paddingLeft: "20px" }}
>
My Logo
</div>
</Col>
<Col xs={0} sm={0} md={20}>
<Menu theme="dark" mode="horizontal" defaultSelectedKeys={["1"]}>
<Menu.Item key="1" icon={<HomeOutlined />}>
Home
</Menu.Item>
<Menu.Item key="2" icon={<UserOutlined />}>
Profile
</Menu.Item>
<Menu.Item key="3" icon={<SettingOutlined />}>
Settings
</Menu.Item>
<Menu.Item key="4">
<Button type="primary" style={{ marginRight: "10px" }}>
Sign in
</Button>
<Button>Sign up</Button>
</Menu.Item>
</Menu>
</Col>
<Col xs={2} sm={2} md={0}>
<Button type="primary" onClick={showDrawer}>
<MenuOutlined />
</Button>
</Col>
</Row>
<Drawer
title="Menu"
placement="right"
onClick={onClose}
onClose={onClose}
visible={visible}
>
<Menu mode="vertical" defaultSelectedKeys={["1"]}>
<Menu.Item key="1" icon={<HomeOutlined />}>
Home
</Menu.Item>
<Menu.Item key="2" icon={<UserOutlined />}>
Profile
</Menu.Item>
<Menu.Item key="3" icon={<SettingOutlined />}>
Settings
</Menu.Item>
<Menu.Item key="4">
<Button type="primary" style={{ marginRight: "10px" }}>
Sign in
</Button>
<Button>Sign up</Button>
</Menu.Item>
</Menu>
</Drawer>
</Header>
</Layout>
);
};
export default ResponsiveNav;
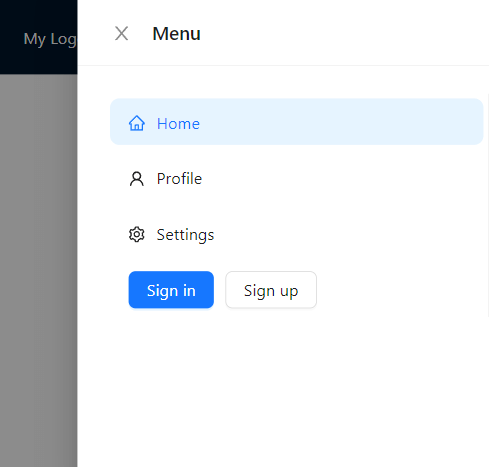