In this tutorial, we will create a search bar in React using Ant Design 5. We will demonstrate how to implement an Ant Design 5 search bar with TypeScript, including an example featuring a search bar with an icon.
install & setup vite + react + typescript + ant design 5
React Ant Design 5 Search Bar Example
1. Create react ant design 5 simple search bar using react-antd Input component.
import { useState } from "react";
import { Input } from "antd";
export default function SearchBar() {
const [value, setValue] = useState("");
const onChange = (event) => {
setValue(event.target.value);
};
const onSearch = (value) => {
console.log(value);
};
return (
<Input.Search
placeholder="input search text"
value={value}
onChange={onChange}
onSearch={onSearch}
enterButton
style={{ width: "300px" }}
/>
);
}

2. React ant design 5 search bar with icons using typescript.
import React from 'react';
import { AudioOutlined } from '@ant-design/icons';
import { Input, Space } from 'antd';
const { Search } = Input;
const suffix = (
<AudioOutlined
style={{
fontSize: 16,
color: '#1890ff',
}}
/>
);
const onSearch = (value: string) => console.log(value);
const App: React.FC = () => (
<Space direction="vertical">
<Search placeholder="input search text" onSearch={onSearch} style={{ width: 200 }} />
<Search placeholder="input search text" allowClear onSearch={onSearch} style={{ width: 200 }} />
<Search
addonBefore="https://"
placeholder="input search text"
allowClear
onSearch={onSearch}
style={{ width: 304 }}
/>
<Search placeholder="input search text" onSearch={onSearch} enterButton />
<Search
placeholder="input search text"
allowClear
enterButton="Search"
size="large"
onSearch={onSearch}
/>
<Search
placeholder="input search text"
enterButton="Search"
size="large"
suffix={suffix}
onSearch={onSearch}
/>
</Space>
);
export default App;
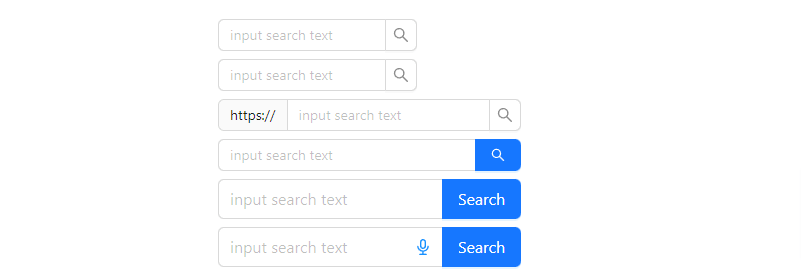
3. React ant design 5 search bar with AutoComplete dropdown.
import { useState } from "react";
import { AutoComplete, Input } from "antd";
export default function SearchBar() {
const [value, setValue] = useState("");
const options = [
{ value: "Option1" },
{ value: "Option2" },
{ value: "Option3" },
];
const onSearch = (searchText) => {
setValue(searchText);
};
const onSelect = (data) => {
console.log("onSelect", data);
};
return (
<AutoComplete
options={options}
style={{
width: 300,
}}
onSelect={onSelect}
onSearch={onSearch}
>
<Input.Search size="large" value={value} enterButton />
</AutoComplete>
);
}