Bootstrap 5 provides powerful tools to build professional-looking login and signup modals that work seamlessly across all devices. This guide will walk you through creating three different modal designs that you can implement in your projects.
Understanding Bootstrap Modals
Bootstrap modals are popup dialogs that provide a clean way to present focused content to users without navigating away from the current page. They’re particularly effective for login and signup forms, as they maintain context while gathering user information.
Essential Components
Before diving into the examples, ensure you include Bootstrap’s required CSS and JavaScript files in your project:
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
Basic Login Modal
The basic login modal provides a clean, straightforward approach suitable for most applications. It focuses on essential functionality without overwhelming users.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Basic Login/Signup Modal</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<!-- Login Modal Button -->
<button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#loginModal">
Login
</button>
<!-- Login Modal -->
<div class="modal fade" id="loginModal" tabindex="-1" aria-labelledby="loginModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="loginModalLabel">Login</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<form>
<div class="mb-3">
<label for="email" class="form-label">Email address</label>
<input type="email" class="form-control" id="email" required>
</div>
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<input type="password" class="form-control" id="password" required>
</div>
<div class="mb-3 form-check">
<input type="checkbox" class="form-check-input" id="rememberMe">
<label class="form-check-label" for="rememberMe">Remember me</label>
</div>
<button type="submit" class="btn btn-primary w-100">Login</button>
</form>
</div>
<div class="modal-footer">
<p class="w-100 text-center mb-0">Don't have an account? <a href="#" data-bs-toggle="modal" data-bs-target="#signupModal">Sign up</a></p>
</div>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
Social Login Integration
Social login options can significantly improve user experience by providing alternative authentication methods. This design combines traditional email login with popular social media options.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Social Login Modal</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0/css/all.min.css">
</head>
<body>
<!-- Login Modal Button -->
<button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#socialLoginModal">
Login with Social
</button>
<!-- Login Modal -->
<div class="modal fade" id="socialLoginModal" tabindex="-1" aria-labelledby="socialLoginModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="socialLoginModalLabel">Login to Your Account</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<div class="d-grid gap-2 mb-4">
<button class="btn btn-outline-dark" type="button">
<i class="fab fa-google me-2"></i>Continue with Google
</button>
<button class="btn btn-outline-primary" type="button">
<i class="fab fa-facebook-f me-2"></i>Continue with Facebook
</button>
</div>
<div class="text-center mb-4">
<span class="bg-white px-2">or</span>
<hr class="mt-n3">
</div>
<form>
<div class="mb-3">
<label for="email" class="form-label">Email address</label>
<input type="email" class="form-control" id="email" required>
</div>
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<input type="password" class="form-control" id="password" required>
<div class="form-text text-end">
<a href="#" class="text-decoration-none">Forgot password?</a>
</div>
</div>
<button type="submit" class="btn btn-primary w-100">Login</button>
</form>
</div>
<div class="modal-footer justify-content-center">
<p class="mb-0">New to our platform? <a href="#" class="text-decoration-none">Create an account</a></p>
</div>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
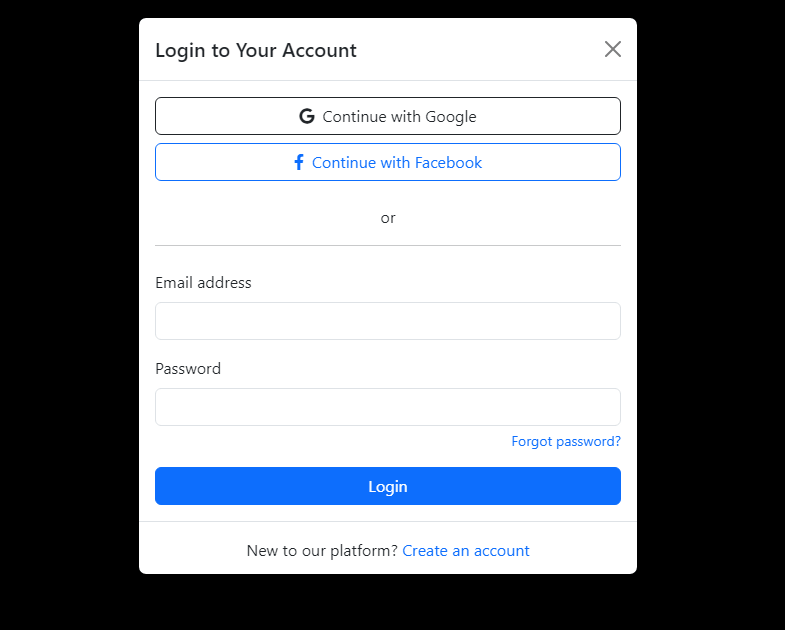
Tabbed Login/Signup Interface
A tabbed interface provides a sophisticated solution that combines both login and registration functions in a single modal, reducing interface clutter and improving user flow.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Tabbed Login/Signup Modal</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<!-- Modal Button -->
<button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#tabbedModal">
Login/Signup
</button>
<!-- Tabbed Modal -->
<div class="modal fade" id="tabbedModal" tabindex="-1" aria-labelledby="tabbedModalLabel" aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<ul class="nav nav-tabs w-100 border-bottom-0" role="tablist">
<li class="nav-item" role="presentation">
<button class="nav-link active" data-bs-toggle="tab" data-bs-target="#loginTab" type="button">Login</button>
</li>
<li class="nav-item" role="presentation">
<button class="nav-link" data-bs-toggle="tab" data-bs-target="#signupTab" type="button">Sign Up</button>
</li>
</ul>
<button type="button" class="btn-close ms-auto" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<div class="tab-content">
<!-- Login Tab -->
<div class="tab-pane fade show active" id="loginTab">
<form>
<div class="mb-3">
<label for="loginEmail" class="form-label">Email address</label>
<input type="email" class="form-control" id="loginEmail" required>
</div>
<div class="mb-3">
<label for="loginPassword" class="form-label">Password</label>
<input type="password" class="form-control" id="loginPassword" required>
</div>
<div class="d-flex justify-content-between mb-3">
<div class="form-check">
<input type="checkbox" class="form-check-input" id="rememberMe">
<label class="form-check-label" for="rememberMe">Remember me</label>
</div>
<a href="#" class="text-decoration-none">Forgot password?</a>
</div>
<button type="submit" class="btn btn-primary w-100">Login</button>
</form>
</div>
<!-- Signup Tab -->
<div class="tab-pane fade" id="signupTab">
<form>
<div class="mb-3">
<label for="signupName" class="form-label">Full Name</label>
<input type="text" class="form-control" id="signupName" required>
</div>
<div class="mb-3">
<label for="signupEmail" class="form-label">Email address</label>
<input type="email" class="form-control" id="signupEmail" required>
</div>
<div class="mb-3">
<label for="signupPassword" class="form-label">Password</label>
<input type="password" class="form-control" id="signupPassword" required>
</div>
<div class="mb-3">
<label for="confirmPassword" class="form-label">Confirm Password</label>
<input type="password" class="form-control" id="confirmPassword" required>
</div>
<div class="mb-3 form-check">
<input type="checkbox" class="form-check-input" id="terms">
<label class="form-check-label" for="terms">I agree to the Terms & Conditions</label>
</div>
<button type="submit" class="btn btn-primary w-100">Create Account</button>
</form>
</div>
</div>
</div>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
Feel free to customize these modal examples to match your project’s requirements and design preferences. The flexibility of Bootstrap 5 allows for extensive customization while maintaining responsive behavior and accessibility standards.