In this tutorial, we will create footer page in react with material ui (mui 5). We will see sticky footer, footer with social media icon example with react material UI 5.
Install & Setup Vite + React + Typescript + MUI 5
React Material UI 5 Footer Example
1. Create react mui 5 simple footer using react-mui Box, Container, Typography, Link component.
import * as React from "react";
import Container from "@mui/material/Container";
import Typography from "@mui/material/Typography";
import Link from "@mui/material/Link";
import { Box } from "@mui/material";
export default function Footer() {
return (
<Box
sx={{
backgroundColor: (theme) =>
theme.palette.mode === "light"
? theme.palette.grey[200]
: theme.palette.grey[800],
p: 6,
}}
component="footer"
>
<Container maxWidth="sm">
<Typography variant="body2" color="text.secondary" align="center">
{"Copyright © "}
<Link color="inherit" href="https://your-website.com/">
Your Website
</Link>{" "}
{new Date().getFullYear()}
{"."}
</Typography>
</Container>
</Box>
);
}

2. react mui 5 sticky footer page.
import * as React from 'react';
import CssBaseline from '@mui/material/CssBaseline';
import { createTheme, ThemeProvider } from '@mui/material/styles';
import Box from '@mui/material/Box';
import Typography from '@mui/material/Typography';
import Container from '@mui/material/Container';
import Link from '@mui/material/Link';
function Copyright() {
return (
<Typography variant="body2" color="text.secondary">
{'Copyright © '}
<Link color="inherit" href="https://mui.com/">
Your Website
</Link>{' '}
{new Date().getFullYear()}
{'.'}
</Typography>
);
}
const defaultTheme = createTheme();
export default function StickyFooter() {
return (
<ThemeProvider theme={defaultTheme}>
<Box
sx={{
display: 'flex',
flexDirection: 'column',
minHeight: '100vh',
}}
>
<CssBaseline />
<Container component="main" sx={{ mt: 8, mb: 2 }} maxWidth="sm">
<Typography variant="h2" component="h1" gutterBottom>
Sticky footer
</Typography>
<Typography variant="h5" component="h2" gutterBottom>
{'Pin a footer to the bottom of the viewport.'}
{'The footer will move as the main element of the page grows.'}
</Typography>
<Typography variant="body1">Sticky footer placeholder.</Typography>
</Container>
<Box
component="footer"
sx={{
py: 3,
px: 2,
mt: 'auto',
backgroundColor: (theme) =>
theme.palette.mode === 'light'
? theme.palette.grey[200]
: theme.palette.grey[800],
}}
>
<Container maxWidth="sm">
<Typography variant="body1">
My sticky footer can be found here.
</Typography>
<Copyright />
</Container>
</Box>
</Box>
</ThemeProvider>
);
}
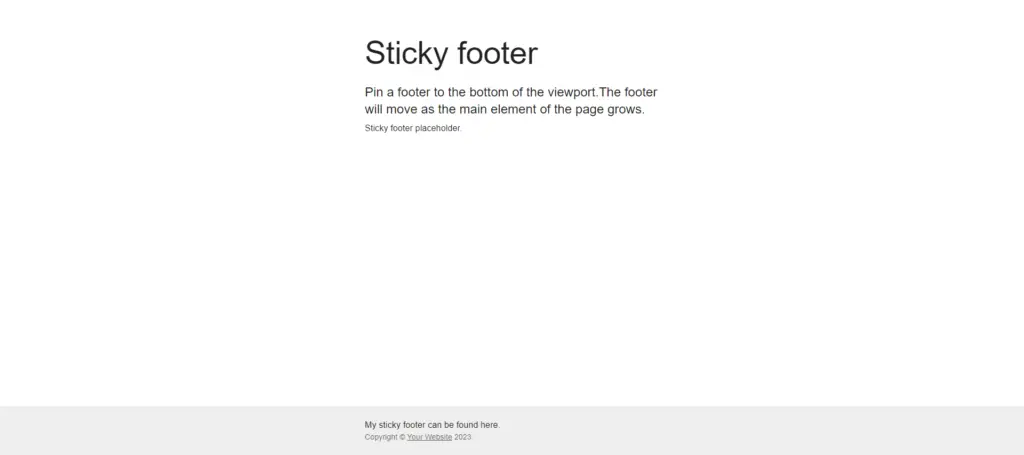
3. react mui 5 footer with social media icon and address.
import * as React from "react";
import Container from "@mui/material/Container";
import Typography from "@mui/material/Typography";
import Link from "@mui/material/Link";
import Grid from "@mui/material/Grid";
import { Facebook, Instagram, Twitter } from "@mui/icons-material";
import { Box } from "@mui/material";
export default function Footer() {
return (
<Box
component="footer"
sx={{
backgroundColor: (theme) =>
theme.palette.mode === "light"
? theme.palette.grey[200]
: theme.palette.grey[800],
p: 6,
}}
>
<Container maxWidth="lg">
<Grid container spacing={5}>
<Grid item xs={12} sm={4}>
<Typography variant="h6" color="text.primary" gutterBottom>
About Us
</Typography>
<Typography variant="body2" color="text.secondary">
We are XYZ company, dedicated to providing the best service to our
customers.
</Typography>
</Grid>
<Grid item xs={12} sm={4}>
<Typography variant="h6" color="text.primary" gutterBottom>
Contact Us
</Typography>
<Typography variant="body2" color="text.secondary">
123 Main Street, Anytown, USA
</Typography>
<Typography variant="body2" color="text.secondary">
Email: [email protected]
</Typography>
<Typography variant="body2" color="text.secondary">
Phone: +1 234 567 8901
</Typography>
</Grid>
<Grid item xs={12} sm={4}>
<Typography variant="h6" color="text.primary" gutterBottom>
Follow Us
</Typography>
<Link href="https://www.facebook.com/" color="inherit">
<Facebook />
</Link>
<Link
href="https://www.instagram.com/"
color="inherit"
sx={{ pl: 1, pr: 1 }}
>
<Instagram />
</Link>
<Link href="https://www.twitter.com/" color="inherit">
<Twitter />
</Link>
</Grid>
</Grid>
<Box mt={5}>
<Typography variant="body2" color="text.secondary" align="center">
{"Copyright © "}
<Link color="inherit" href="https://your-website.com/">
Your Website
</Link>{" "}
{new Date().getFullYear()}
{"."}
</Typography>
</Box>
</Container>
</Box>
);
}
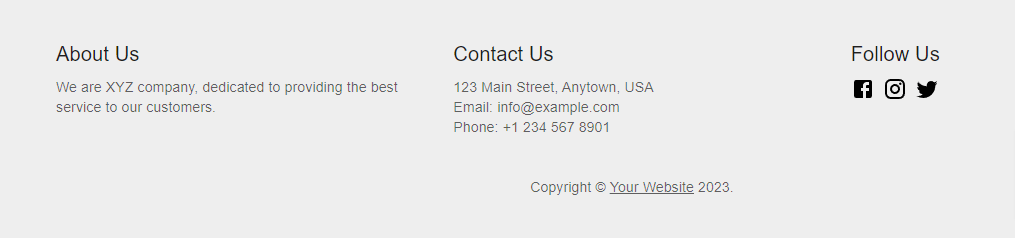
4. Build a responsive footer section using React MUI 5 and TypeScript.
import * as React from 'react';
import { Box, Grid, Link, Typography, Container, IconButton } from '@mui/material';
import FacebookIcon from '@mui/icons-material/Facebook';
import TwitterIcon from '@mui/icons-material/Twitter';
import InstagramIcon from '@mui/icons-material/Instagram';
// Replace these with your own social media URLs
const socialMediaLinks = {
facebook: '#',
twitter: '#',
instagram: '#',
};
const Footer: React.FC = () => {
return (
<Box
sx={{
bgcolor: 'background.paper',
color: 'text.secondary',
py: 3,
borderTop: '1px solid',
borderColor: 'divider',
}}
>
<Container maxWidth={false}>
<Grid container spacing={2} justifyContent="space-between">
<Grid item xs={12} sm={6} md={3}>
<Typography variant="h6" color="text.primary" gutterBottom>
Brand Name
</Typography>
{/* Add your logo component or image here */}
</Grid>
<Grid item xs={6} sm={3} md={2}>
<Typography variant="subtitle1" color="text.primary" gutterBottom>
PRODUCT
</Typography>
<Link href="#" color="inherit" display="block">Features</Link>
<Link href="#" color="inherit" display="block">Integrations</Link>
<Link href="#" color="inherit" display="block">Pricing</Link>
<Link href="#" color="inherit" display="block">FAQ</Link>
</Grid>
<Grid item xs={6} sm={3} md={2}>
<Typography variant="subtitle1" color="text.primary" gutterBottom>
COMPANY
</Typography>
<Link href="#" color="inherit" display="block">About Us</Link>
<Link href="#" color="inherit" display="block">Careers</Link>
<Link href="#" color="inherit" display="block">Privacy Policy</Link>
<Link href="#" color="inherit" display="block">Terms of Service</Link>
</Grid>
<Grid item xs={6} sm={3} md={2}>
<Typography variant="subtitle1" color="text.primary" gutterBottom>
DEVELOPERS
</Typography>
<Link href="#" color="inherit" display="block">Public API</Link>
<Link href="#" color="inherit" display="block">Documentation</Link>
<Link href="#" color="inherit" display="block">Guides</Link>
</Grid>
<Grid item xs={6} sm={3} md={2}>
<Typography variant="subtitle1" color="text.primary" gutterBottom>
SOCIAL MEDIA
</Typography>
<IconButton aria-label="Facebook" color="inherit" component="a" href={socialMediaLinks.facebook}>
<FacebookIcon />
</IconButton>
<IconButton aria-label="Twitter" color="inherit" component="a" href={socialMediaLinks.twitter}>
<TwitterIcon />
</IconButton>
<IconButton aria-label="Instagram" color="inherit" component="a" href={socialMediaLinks.instagram}>
<InstagramIcon />
</IconButton>
</Grid>
</Grid>
<Typography variant="body2" color="text.secondary" align="center" sx={{ pt: 4 }}>
© 2024 Company Co. All rights reserved.
</Typography>
</Container>
</Box>
);
};
export default Footer;
