In this tutorial, we’ll show you how to create a footer section in a Next.js application using the ShadCN UI library.
Before create footer in next js with shadcn ui you need to install lucide icon.
npm install lucide-react
1. Create next js with shadcn ui responsive footer using Facebook, Instagram, Linkedin, Twitter lucide icon.
import Link from "next/link"
import { Facebook, Instagram, Linkedin, Twitter } from "lucide-react"
export default function FooterDemo() {
return (
<footer className="bg-gray-200">
<div className="container flex flex-wrap items-center justify-center px-4 py-8 mx-auto lg:justify-between">
<div className="flex flex-wrap justify-center">
<ul className="flex items-center space-x-4">
<li><Link href={"/"}>Home</Link></li>
<li><Link href={"/about"}>About</Link></li>
<li><Link href={"/contact"}>Contact US</Link></li>
<li><Link href={"/terms"}>Terms & Condition</Link></li>
</ul>
</div>
<div className="flex justify-center space-x-4 mt-4 lg:mt-0">
<Link href={""}>
<Facebook />
</Link>
<Link href={""}>
<Twitter />
</Link>
<Link href={""}>
<Instagram />
</Link>
<Link href={""}>
<Linkedin />
</Link>
</div>
</div>
<div className="pb-2">
<p className="text-center">
@2024 All rights reserved by your website.
</p>
</div>
</footer>
)
}

2. Next js with shadcn ui footer section with links logo, social media icon.
import Link from "next/link"
import { Facebook, Instagram, Linkedin, Twitter } from "lucide-react"
import { Button } from "@/components/ui/button"
import { Input } from "@/components/ui/input"
export default function FooterDemo() {
return (
<footer className="bg-gray-900">
<div
className="
container
flex flex-col flex-wrap
px-4
py-16
mx-auto
md:items-center
lg:items-start
md:flex-row md:flex-nowrap
"
>
<div className="flex-shrink-0 w-64 mx-auto text-center md:mx-0 md:text-left">
<Link href={"/"} className="text-2xl text-white">
My Website
</Link>
<p className="mt-2 text-xs text-justify text-gray-400">
Footer is a valuable resource that complements the main content of
the website by providing quick links, legal information, and ways to
connect, creating a well-rounded and user-friendly experience for
visitors.
</p>
<div className="flex mt-4">
<Input type="email" placeholder="Email" />
<Button variant="destructive">Subscribe</Button>
</div>
<div className="flex justify-center mt-4 space-x-4 lg:mt-2">
<Link href={""}>
<Facebook className="text-blue-500" />
</Link>
<Link href={""}>
<Twitter className="text-sky-300" />
</Link>
<Link href={""}>
<Instagram className="text-pink-500" />
</Link>
<Link href={""}>
<Linkedin className="text-blue-400" />
</Link>
</div>
</div>
<div className="justify-between w-full mt-4 text-center lg:flex">
<div className="w-full px-4 lg:w-1/3 md:w-1/2">
<h2 className="mb-2 font-bold tracking-widest text-gray-100">
Quick Links
</h2>
<ul className="mb-8 space-y-2 text-sm list-none">
<li>
<Link href={"/"} className="text-gray-300">
Link 1
</Link>
</li>
<li>
<Link href={"/"} className="text-gray-300">
Link 2
</Link>
</li>
<li>
<Link href={"/"} className="text-gray-300">
Link 3
</Link>
</li>
<li>
<Link href={"/"} className="text-gray-300">
Link 4
</Link>
</li>
</ul>
</div>
<div className="w-full px-4 lg:w-1/3 md:w-1/2">
<h2 className="mb-2 font-bold tracking-widest text-gray-100">
Quick Links
</h2>
<ul className="mb-8 space-y-2 text-sm list-none">
<li>
<Link href={"/"} className="text-gray-300">
Link 1
</Link>
</li>
<li>
<Link href={"/"} className="text-gray-300">
Link 2
</Link>
</li>
<li>
<Link href={"/"} className="text-gray-300">
Link 3
</Link>
</li>
<li>
<Link href={"/"} className="text-gray-300">
Link 4
</Link>
</li>
</ul>
</div>
<div className="w-full px-4 lg:w-1/3 md:w-1/2">
<h2 className="mb-2 font-bold tracking-widest text-gray-100">
Quick Links
</h2>
<ul className="mb-8 space-y-2 text-sm list-none">
<li>
<Link href={"/"} className="text-gray-300">
Link 1
</Link>
</li>
<li>
<Link href={"/"} className="text-gray-300">
Link 2
</Link>
</li>
<li>
<Link href={"/"} className="text-gray-300">
Link 3
</Link>
</li>
<li>
<Link href={"/"} className="text-gray-300">
Link 4
</Link>
</li>
</ul>
</div>
</div>
</div>
<div className="flex justify-center -mt-12">
<p className="text-center text-white pb-2">
@2024 All rights reserved by your website.
</p>
</div>
</footer>
)
}
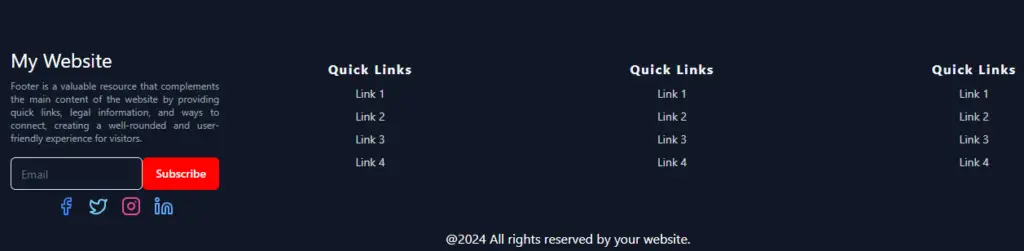