Radio buttons are essential form elements that allow users to select a single option from multiple choices. Bootstrap 5 provides powerful tools to transform traditional radio buttons into modern, user-friendly interface elements. This guide explores different approaches to implementing radio buttons and shares practical examples for your web projects.
Understanding Bootstrap Radio Buttons
Radio buttons in Bootstrap 5 offer significant flexibility in terms of styling and functionality. Whether you need simple form inputs or complex interactive elements, Bootstrap’s utility classes and components provide the foundation for creating engaging user experiences.
Basic Implementation
The foundation of Bootstrap radio buttons starts with the .form-check
class. Here’s the basic structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Basic Radio Buttons</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body class="p-4">
<div class="container">
<h5>Select Your Preferred Contact Method</h5>
<!-- Basic Radio Buttons -->
<div class="form-check mb-2">
<input class="form-check-input" type="radio" name="contactMethod" id="emailRadio" checked>
<label class="form-check-label" for="emailRadio">
Email
</label>
</div>
<div class="form-check mb-2">
<input class="form-check-input" type="radio" name="contactMethod" id="phoneRadio">
<label class="form-check-label" for="phoneRadio">
Phone
</label>
</div>
<div class="form-check mb-4">
<input class="form-check-input" type="radio" name="contactMethod" id="smsRadio">
<label class="form-check-label" for="smsRadio">
SMS
</label>
</div>
<!-- Disabled Radio Buttons -->
<h5 class="mt-4">Disabled Options</h5>
<div class="form-check mb-2">
<input class="form-check-input" type="radio" name="disabledGroup" id="disabledRadio1" disabled>
<label class="form-check-label" for="disabledRadio1">
Disabled option
</label>
</div>
<div class="form-check">
<input class="form-check-input" type="radio" name="disabledGroup" id="disabledRadio2" checked disabled>
<label class="form-check-label" for="disabledRadio2">
Disabled checked option
</label>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
Key considerations for basic implementation:
- Always use the same
name
attribute for related radio buttons - Each input requires a unique
id
that matches its label’sfor
attribute - Include the
.form-check-input
and.form-check-label
classes
Creating Interactive Card Radio Buttons
For more engaging interfaces, transform radio buttons into interactive cards. This approach works particularly well for pricing plans or feature selection:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Radio Button Cards</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<style>
.radio-card {
cursor: pointer;
border: 2px solid #dee2e6;
transition: all 0.3s ease;
}
.radio-card:hover {
border-color: #0d6efd;
}
.radio-input:checked + .radio-card {
border-color: #0d6efd;
background-color: #f8f9ff;
}
</style>
</head>
<body class="p-4">
<div class="container">
<h5 class="mb-4">Select Your Subscription Plan</h5>
<div class="row g-4">
<!-- Basic Plan -->
<div class="col-md-4">
<input type="radio" class="radio-input d-none" name="subscription" id="basicPlan" checked>
<label class="radio-card card h-100 p-4" for="basicPlan">
<div class="card-body p-0">
<h5 class="card-title">Basic Plan</h5>
<h2 class="card-text mb-3">$9.99</h2>
<ul class="list-unstyled">
<li class="mb-2">✓ 10GB Storage</li>
<li class="mb-2">✓ Basic Support</li>
<li>✓ 1 User</li>
</ul>
</div>
</label>
</div>
<!-- Pro Plan -->
<div class="col-md-4">
<input type="radio" class="radio-input d-none" name="subscription" id="proPlan">
<label class="radio-card card h-100 p-4" for="proPlan">
<div class="card-body p-0">
<h5 class="card-title">Pro Plan</h5>
<h2 class="card-text mb-3">$19.99</h2>
<ul class="list-unstyled">
<li class="mb-2">✓ 50GB Storage</li>
<li class="mb-2">✓ Priority Support</li>
<li>✓ 5 Users</li>
</ul>
</div>
</label>
</div>
<!-- Enterprise Plan -->
<div class="col-md-4">
<input type="radio" class="radio-input d-none" name="subscription" id="enterprisePlan">
<label class="radio-card card h-100 p-4" for="enterprisePlan">
<div class="card-body p-0">
<h5 class="card-title">Enterprise Plan</h5>
<h2 class="card-text mb-3">$49.99</h2>
<ul class="list-unstyled">
<li class="mb-2">✓ Unlimited Storage</li>
<li class="mb-2">✓ 24/7 Support</li>
<li>✓ Unlimited Users</li>
</ul>
</div>
</label>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
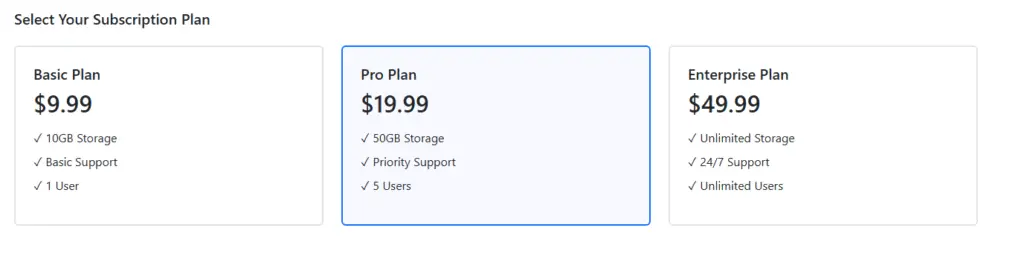
Button Group Radio Implementation
Bootstrap’s button groups offer another stylish approach to radio buttons:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Radio Button Groups</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0/css/all.min.css">
</head>
<body class="p-4">
<div class="container">
<h5 class="mb-4">Payment Method</h5>
<!-- Button Group Radio -->
<div class="btn-group w-100 mb-4" role="group" aria-label="Payment method selection">
<input type="radio" class="btn-check" name="paymentMethod" id="creditCard" checked>
<label class="btn btn-outline-primary" for="creditCard">
<i class="fas fa-credit-card me-2"></i>Credit Card
</label>
<input type="radio" class="btn-check" name="paymentMethod" id="paypal">
<label class="btn btn-outline-primary" for="paypal">
<i class="fab fa-paypal me-2"></i>PayPal
</label>
<input type="radio" class="btn-check" name="paymentMethod" id="bankTransfer">
<label class="btn btn-outline-primary" for="bankTransfer">
<i class="fas fa-university me-2"></i>Bank Transfer
</label>
</div>
<!-- Vertical Button Group -->
<h5 class="mb-3">Delivery Options</h5>
<div class="btn-group-vertical w-100" role="group" aria-label="Delivery options">
<input type="radio" class="btn-check" name="deliveryOption" id="standard" checked>
<label class="btn btn-outline-success text-start" for="standard">
<i class="fas fa-truck me-2"></i>
<span class="d-flex flex-column">
<strong>Standard Delivery</strong>
<small class="text-muted">3-5 business days</small>
</span>
</label>
<input type="radio" class="btn-check" name="deliveryOption" id="express">
<label class="btn btn-outline-success text-start" for="express">
<i class="fas fa-shipping-fast me-2"></i>
<span class="d-flex flex-column">
<strong>Express Delivery</strong>
<small class="text-muted">1-2 business days</small>
</span>
</label>
<input type="radio" class="btn-check" name="deliveryOption" id="overnight">
<label class="btn btn-outline-success text-start" for="overnight">
<i class="fas fa-meteor me-2"></i>
<span class="d-flex flex-column">
<strong>Overnight Delivery</strong>
<small class="text-muted">Next business day</small>
</span>
</label>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>