In this tutorial, we’ll create a responsive navbar menu in Next.js with Shadcn UI. First, you need to set up a Next.js project with Shadcn UI.
Before creating a responsive navbar in Next.js 13 with Shadcn UI, you need to install the Lucide icon library.
npm install lucide-react
1. Create a responsive navbar in Next.js 13 with Shadcn UI, incorporating a hamburger menu icon using the Menu Lucide icon.
"use client"
import * as React from "react"
import Link from "next/link"
import { Menu } from "lucide-react"
export default function Navbar() {
const [state, setState] = React.useState(false)
const menus = [
{ title: "Home", path: "/your-path" },
{ title: "Blog", path: "/your-path" },
{ title: "About Us", path: "/your-path" },
{ title: "Contact Us", path: "/your-path" },
]
return (
<nav className="bg-white w-full border-b md:border-0">
<div className="items-center px-4 max-w-screen-xl mx-auto md:flex md:px-8">
<div className="flex items-center justify-between py-3 md:py-5 md:block">
<Link href="/">
<h1 className="text-3xl font-bold text-purple-600">Logo</h1>
</Link>
<div className="md:hidden">
<button
className="text-gray-700 outline-none p-2 rounded-md focus:border-gray-400 focus:border"
onClick={() => setState(!state)}
>
<Menu />
</button>
</div>
</div>
<div
className={`flex-1 justify-self-center pb-3 mt-8 md:block md:pb-0 md:mt-0 ${
state ? "block" : "hidden"
}`}
>
<ul className="justify-center items-center space-y-8 md:flex md:space-x-6 md:space-y-0">
{menus.map((item, idx) => (
<li key={idx} className="text-gray-600 hover:text-indigo-600">
<Link href={item.path}>{item.title}</Link>
</li>
))}
</ul>
</div>
</div>
</nav>
)
}
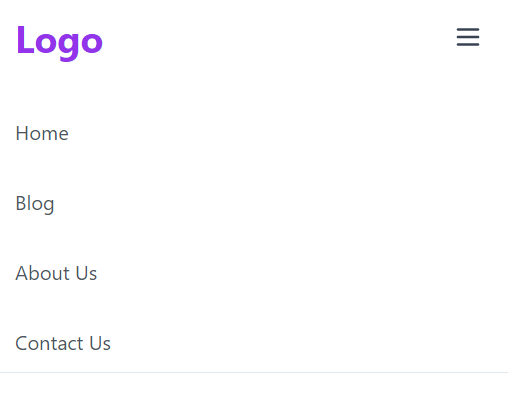
2. Create a responsive navbar in Next.js 13 with Shadcn UI, featuring a search bar using the Menu and Search Lucide icons, along with the useState
hook.
"use client"
import * as React from "react"
import Link from "next/link"
import { Menu, Search } from "lucide-react"
export default function Navbar() {
const [state, setState] = React.useState(false)
const menus = [
{ title: "Home", path: "/your-path" },
{ title: "Blog", path: "/your-path" },
{ title: "About Us", path: "/your-path" },
{ title: "Contact Us", path: "/your-path" },
]
return (
<nav className="bg-white w-full border-b md:border-0">
<div className="items-center px-4 max-w-screen-xl mx-auto md:flex md:px-8">
<div className="flex items-center justify-between py-3 md:py-5 md:block">
<Link href="/">
<h1 className="text-3xl font-bold text-purple-600">Logo</h1>
</Link>
<div className="md:hidden">
<button
className="text-gray-700 outline-none p-2 rounded-md focus:border-gray-400 focus:border"
onClick={() => setState(!state)}
>
<Menu />
</button>
</div>
</div>
<div
className={`flex-1 justify-self-center pb-3 mt-8 md:block md:pb-0 md:mt-0 ${
state ? "block" : "hidden"
}`}
>
<ul className="justify-center items-center space-y-8 md:flex md:space-x-6 md:space-y-0">
{menus.map((item, idx) => (
<li key={idx} className="text-gray-600 hover:text-indigo-600">
<Link href={item.path}>{item.title}</Link>
</li>
))}
<form className="flex items-center space-x-2 border rounded-md p-2">
<Search className="h-5 w-5 flex-none text-gray-300" />
<input
className="w-full outline-none appearance-none placeholder-gray-500 text-gray-500 sm:w-auto"
type="text"
placeholder="Search"
/>
</form>
</ul>
</div>
</div>
</nav>
)
}

Sources
- shadcn/ui Input (ui.shadcn.com/docs)
- nextjs link components (nextjs.org/docs)
- lucide icon (lucide.dev/icons)