Creating a responsive navigation menu is essential for a seamless user experience. In this tutorial, we’ll build a stylish menu using Shadcn UI with Next.js, ensuring adaptability across devices. Follow along to enhance your Next.js projects with a sleek, responsive navigation solution.
How to Install Shadcn UI in Next.js Project
Create a responsive Navigation Menu using sheet and button components. Before you start, you need to install them.
npx shadcn-ui@latest add button
npx shadcn-ui@latest add sheet
export default function Demo() {
return (
<header className="flex h-20 w-full shrink-0 items-center px-4 md:px-6">
<Sheet>
<SheetTrigger asChild>
<Button variant="outline" size="icon" className="lg:hidden">
<MenuIcon className="h-6 w-6" />
<span className="sr-only">Toggle navigation menu</span>
</Button>
</SheetTrigger>
<SheetContent side="left">
<Link href="#" className="flex items-center gap-2" prefetch={false}>
<MountainIcon className="h-6 w-6" />
<span className="text-lg font-semibold">Acme Inc</span>
</Link>
<div className="grid gap-4 py-6">
<Link href="#" className="flex w-full items-center py-2 text-lg font-semibold" prefetch={false}>
Home
</Link>
<Link href="#" className="flex w-full items-center py-2 text-lg font-semibold" prefetch={false}>
About
</Link>
<Link href="#" className="flex w-full items-center py-2 text-lg font-semibold" prefetch={false}>
Services
</Link>
<Link href="#" className="flex w-full items-center py-2 text-lg font-semibold" prefetch={false}>
Contact
</Link>
</div>
</SheetContent>
</Sheet>
<div className="ml-auto hidden lg:flex">
<nav className="flex items-center gap-6">
<Link href="#" className="text-sm font-medium hover:underline underline-offset-4" prefetch={false}>
Home
</Link>
<Link href="#" className="text-sm font-medium hover:underline underline-offset-4" prefetch={false}>
About
</Link>
<Link href="#" className="text-sm font-medium hover:underline underline-offset-4" prefetch={false}>
Services
</Link>
<Link href="#" className="text-sm font-medium hover:underline underline-offset-4" prefetch={false}>
Contact
</Link>
</nav>
<div className="ml-6">
<Button>Get Started</Button>
</div>
</div>
</header>
)
}
function MenuIcon(props) {
return (
<svg
{...props}
xmlns="http://www.w3.org/2000/svg"
width="24"
height="24"
viewBox="0 0 24 24"
fill="none"
stroke="currentColor"
strokeWidth="2"
strokeLinecap="round"
strokeLinejoin="round"
>
<line x1="4" x2="20" y1="12" y2="12" />
<line x1="4" x2="20" y1="6" y2="6" />
<line x1="4" x2="20" y1="18" y2="18" />
</svg>
)
}
function MountainIcon(props) {
return (
<svg
{...props}
xmlns="http://www.w3.org/2000/svg"
width="24"
height="24"
viewBox="0 0 24 24"
fill="none"
stroke="currentColor"
strokeWidth="2"
strokeLinecap="round"
strokeLinejoin="round"
>
<path d="m8 3 4 8 5-5 5 15H2L8 3z" />
</svg>
)
}
function XIcon(props) {
return (
<svg
{...props}
xmlns="http://www.w3.org/2000/svg"
width="24"
height="24"
viewBox="0 0 24 24"
fill="none"
stroke="currentColor"
strokeWidth="2"
strokeLinecap="round"
strokeLinejoin="round"
>
<path d="M18 6 6 18" />
<path d="m6 6 12 12" />
</svg>
)
}
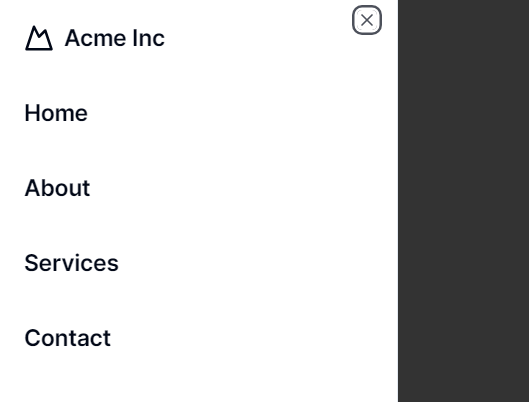
Create a responsive Navigation Menu with Dropdown menu list using sheet, button. collapsible, navigation-menu components. Before you start, you need to install them.
npx shadcn-ui@latest add button
npx shadcn-ui@latest add sheet
npx shadcn-ui@latest add collapsible
npx shadcn-ui@latest add navigation-menu
import { Sheet, SheetTrigger, SheetContent } from "@/components/ui/sheet"
import { Button } from "@/components/ui/button"
import Link from "next/link"
import { Collapsible, CollapsibleTrigger, CollapsibleContent } from "@/components/ui/collapsible"
import { NavigationMenu, NavigationMenuList, NavigationMenuLink, NavigationMenuItem, NavigationMenuTrigger, NavigationMenuContent } from "@/components/ui/navigation-menu"
export default function Demo() {
return (
<header className="flex h-20 w-full shrink-0 items-center px-4 md:px-6">
<Sheet>
<SheetTrigger asChild>
<Button variant="outline" size="icon" className="lg:hidden">
<MenuIcon className="h-6 w-6" />
<span className="sr-only">Toggle navigation menu</span>
</Button>
</SheetTrigger>
<SheetContent side="left">
<Link href="#" prefetch={false}>
<MountainIcon className="h-6 w-6" />
<span className="sr-only">Acme Inc</span>
</Link>
<div className="grid gap-2 py-6">
<Link href="#" className="flex w-full items-center py-2 text-lg font-semibold" prefetch={false}>
About
</Link>
<Collapsible className="grid gap-4">
<CollapsibleTrigger className="flex w-full items-center text-lg font-semibold [&[data-state=open]>svg]:rotate-90">
Features <ChevronRightIcon className="ml-auto h-5 w-5 transition-all" />
</CollapsibleTrigger>
<CollapsibleContent>
<div className="-mx-6 grid gap-6 bg-muted p-6">
<Link href="#" className="group grid h-auto w-full justify-start gap-1" prefetch={false}>
<div className="text-sm font-medium leading-none group-hover:underline">Analytics</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Upgrade your reporting with advanced analytics.
</div>
</Link>
<Link href="#" className="group grid h-auto w-full justify-start gap-1" prefetch={false}>
<div className="text-sm font-medium leading-none group-hover:underline">Developer Tools</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Extend your application with our developer tools.
</div>
</Link>
<Link href="#" className="group grid h-auto w-full justify-start gap-1" prefetch={false}>
<div className="text-sm font-medium leading-none group-hover:underline">
Security & Compliance
</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Keep your data secure with our security features.
</div>
</Link>
<Link href="#" className="group grid h-auto w-full justify-start gap-1" prefetch={false}>
<div className="text-sm font-medium leading-none group-hover:underline">Scalability</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Scale your application with our infrastructure.
</div>
</Link>
</div>
</CollapsibleContent>
</Collapsible>
<Link href="#" className="flex w-full items-center py-2 text-lg font-semibold" prefetch={false}>
Pricing
</Link>
<Collapsible className="grid gap-4">
<CollapsibleTrigger className="flex w-full items-center text-lg font-semibold [&[data-state=open]>svg]:rotate-90">
Resources <ChevronRightIcon className="ml-auto h-5 w-5 transition-all" />
</CollapsibleTrigger>
<CollapsibleContent>
<div className="-mx-6 grid gap-6 bg-muted p-6">
<Link href="#" className="group grid h-auto w-full justify-start gap-1" prefetch={false}>
<div className="text-sm font-medium leading-none group-hover:underline">Blog Posts</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Read our latest blog posts.
</div>
</Link>
<Link href="#" className="group grid h-auto w-full justify-start gap-1" prefetch={false}>
<div className="text-sm font-medium leading-none group-hover:underline">Case Studies</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Read our customer case studies.
</div>
</Link>
<Link href="#" className="group grid h-auto w-full justify-start gap-1" prefetch={false}>
<div className="text-sm font-medium leading-none group-hover:underline">Documentation</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Learn how to use our product.
</div>
</Link>
<Link href="#" className="group grid h-auto w-full justify-start gap-1" prefetch={false}>
<div className="text-sm font-medium leading-none group-hover:underline">Help Center</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Get help with our product.
</div>
</Link>
</div>
</CollapsibleContent>
</Collapsible>
<Link href="#" className="flex w-full items-center py-2 text-lg font-semibold" prefetch={false}>
Contact
</Link>
</div>
</SheetContent>
</Sheet>
<Link href="#" className="mr-6 hidden lg:flex" prefetch={false}>
<MountainIcon className="h-6 w-6" />
<span className="sr-only">Acme Inc</span>
</Link>
<NavigationMenu className="hidden lg:flex">
<NavigationMenuList>
<NavigationMenuLink asChild>
<Link
href="#"
className="group inline-flex h-9 w-max items-center justify-center rounded-md bg-background px-4 py-2 text-sm font-medium transition-colors hover:bg-accent hover:text-accent-foreground focus:bg-accent focus:text-accent-foreground focus:outline-none disabled:pointer-events-none disabled:opacity-50 data-[active]:bg-accent/50 data-[state=open]:bg-accent/50"
prefetch={false}
>
About
</Link>
</NavigationMenuLink>
<NavigationMenuItem>
<NavigationMenuTrigger>Features</NavigationMenuTrigger>
<NavigationMenuContent>
<div className="grid w-[400px] p-2">
<NavigationMenuLink asChild>
<Link
href="#"
className="group grid h-auto w-full items-center justify-start gap-1 rounded-md bg-background p-4 text-sm font-medium transition-colors hover:bg-accent hover:text-accent-foreground focus:bg-accent focus:text-accent-foreground focus:outline-none disabled:pointer-events-none disabled:opacity-50 data-[active]:bg-accent/50 data-[state=open]:bg-accent/50"
prefetch={false}
>
<div className="text-sm font-medium leading-none group-hover:underline">Analytics</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Upgrade your reporting with advanced analytics.
</div>
</Link>
</NavigationMenuLink>
<NavigationMenuLink asChild>
<Link
href="#"
className="group grid h-auto w-full items-center justify-start gap-1 rounded-md bg-background p-4 text-sm font-medium transition-colors hover:bg-accent hover:text-accent-foreground focus:bg-accent focus:text-accent-foreground focus:outline-none disabled:pointer-events-none disabled:opacity-50 data-[active]:bg-accent/50 data-[state=open]:bg-accent/50"
prefetch={false}
>
<div className="text-sm font-medium leading-none group-hover:underline">Developer Tools</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Extend your application with our developer tools.
</div>
</Link>
</NavigationMenuLink>
<NavigationMenuLink asChild>
<Link
href="#"
className="group grid h-auto w-full items-center justify-start gap-1 rounded-md bg-background p-4 text-sm font-medium transition-colors hover:bg-accent hover:text-accent-foreground focus:bg-accent focus:text-accent-foreground focus:outline-none disabled:pointer-events-none disabled:opacity-50 data-[active]:bg-accent/50 data-[state=open]:bg-accent/50"
prefetch={false}
>
<div className="text-sm font-medium leading-none group-hover:underline">
Security & Compliance
</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Keep your data secure with our security features.
</div>
</Link>
</NavigationMenuLink>
<NavigationMenuLink asChild>
<Link
href="#"
className="group grid h-auto w-full items-center justify-start gap-1 rounded-md bg-background p-4 text-sm font-medium transition-colors hover:bg-accent hover:text-accent-foreground focus:bg-accent focus:text-accent-foreground focus:outline-none disabled:pointer-events-none disabled:opacity-50 data-[active]:bg-accent/50 data-[state=open]:bg-accent/50"
prefetch={false}
>
<div className="text-sm font-medium leading-none group-hover:underline">Scalability</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Scale your application with our infrastructure.
</div>
</Link>
</NavigationMenuLink>
</div>
</NavigationMenuContent>
</NavigationMenuItem>
<NavigationMenuLink asChild>
<Link
href="#"
className="group inline-flex h-9 w-max items-center justify-center rounded-md bg-background px-4 py-2 text-sm font-medium transition-colors hover:bg-accent hover:text-accent-foreground focus:bg-accent focus:text-accent-foreground focus:outline-none disabled:pointer-events-none disabled:opacity-50 data-[active]:bg-accent/50 data-[state=open]:bg-accent/50"
prefetch={false}
>
Pricing
</Link>
</NavigationMenuLink>
<NavigationMenuItem>
<NavigationMenuTrigger>Resources</NavigationMenuTrigger>
<NavigationMenuContent>
<div className="grid w-[550px] grid-cols-2 p-2">
<NavigationMenuLink asChild>
<Link
href="#"
className="group grid h-auto w-full items-center justify-start gap-1 rounded-md bg-background p-4 text-sm font-medium transition-colors hover:bg-accent hover:text-accent-foreground focus:bg-accent focus:text-accent-foreground focus:outline-none disabled:pointer-events-none disabled:opacity-50 data-[active]:bg-accent/50 data-[state=open]:bg-accent/50"
prefetch={false}
>
<div className="text-sm font-medium leading-none group-hover:underline">Blog Posts</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Read our latest blog posts.
</div>
</Link>
</NavigationMenuLink>
<NavigationMenuLink asChild>
<Link
href="#"
className="group grid h-auto w-full items-center justify-start gap-1 rounded-md bg-background p-4 text-sm font-medium transition-colors hover:bg-accent hover:text-accent-foreground focus:bg-accent focus:text-accent-foreground focus:outline-none disabled:pointer-events-none disabled:opacity-50 data-[active]:bg-accent/50 data-[state=open]:bg-accent/50"
prefetch={false}
>
<div className="text-sm font-medium leading-none group-hover:underline">Case Studies</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Read our customer case studies.
</div>
</Link>
</NavigationMenuLink>
<NavigationMenuLink asChild>
<Link
href="#"
className="group grid h-auto w-full items-center justify-start gap-1 rounded-md bg-background p-4 text-sm font-medium transition-colors hover:bg-accent hover:text-accent-foreground focus:bg-accent focus:text-accent-foreground focus:outline-none disabled:pointer-events-none disabled:opacity-50 data-[active]:bg-accent/50 data-[state=open]:bg-accent/50"
prefetch={false}
>
<div className="text-sm font-medium leading-none group-hover:underline">Documentation</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Learn how to use our product.
</div>
</Link>
</NavigationMenuLink>
<NavigationMenuLink asChild>
<Link
href="#"
className="group grid h-auto w-full items-center justify-start gap-1 rounded-md bg-background p-4 text-sm font-medium transition-colors hover:bg-accent hover:text-accent-foreground focus:bg-accent focus:text-accent-foreground focus:outline-none disabled:pointer-events-none disabled:opacity-50 data-[active]:bg-accent/50 data-[state=open]:bg-accent/50"
prefetch={false}
>
<div className="text-sm font-medium leading-none group-hover:underline">Help Center</div>
<div className="line-clamp-2 text-sm leading-snug text-muted-foreground">
Get help with our product.
</div>
</Link>
</NavigationMenuLink>
</div>
</NavigationMenuContent>
</NavigationMenuItem>
<NavigationMenuLink asChild>
<Link
href="#"
className="group inline-flex h-9 w-max items-center justify-center rounded-md bg-background px-4 py-2 text-sm font-medium transition-colors hover:bg-accent hover:text-accent-foreground focus:bg-accent focus:text-accent-foreground focus:outline-none disabled:pointer-events-none disabled:opacity-50 data-[active]:bg-accent/50 data-[state=open]:bg-accent/50"
prefetch={false}
>
Contact
</Link>
</NavigationMenuLink>
</NavigationMenuList>
</NavigationMenu>
</header>
)
}
function ChevronRightIcon(props) {
return (
<svg
{...props}
xmlns="http://www.w3.org/2000/svg"
width="24"
height="24"
viewBox="0 0 24 24"
fill="none"
stroke="currentColor"
strokeWidth="2"
strokeLinecap="round"
strokeLinejoin="round"
>
<path d="m9 18 6-6-6-6" />
</svg>
)
}
function MenuIcon(props) {
return (
<svg
{...props}
xmlns="http://www.w3.org/2000/svg"
width="24"
height="24"
viewBox="0 0 24 24"
fill="none"
stroke="currentColor"
strokeWidth="2"
strokeLinecap="round"
strokeLinejoin="round"
>
<line x1="4" x2="20" y1="12" y2="12" />
<line x1="4" x2="20" y1="6" y2="6" />
<line x1="4" x2="20" y1="18" y2="18" />
</svg>
)
}
function MountainIcon(props) {
return (
<svg
{...props}
xmlns="http://www.w3.org/2000/svg"
width="24"
height="24"
viewBox="0 0 24 24"
fill="none"
stroke="currentColor"
strokeWidth="2"
strokeLinecap="round"
strokeLinejoin="round"
>
<path d="m8 3 4 8 5-5 5 15H2L8 3z" />
</svg>
)
}
function XIcon(props) {
return (
<svg
{...props}
xmlns="http://www.w3.org/2000/svg"
width="24"
height="24"
viewBox="0 0 24 24"
fill="none"
stroke="currentColor"
strokeWidth="2"
strokeLinecap="round"
strokeLinejoin="round"
>
<path d="M18 6 6 18" />
<path d="m6 6 12 12" />
</svg>
)
}
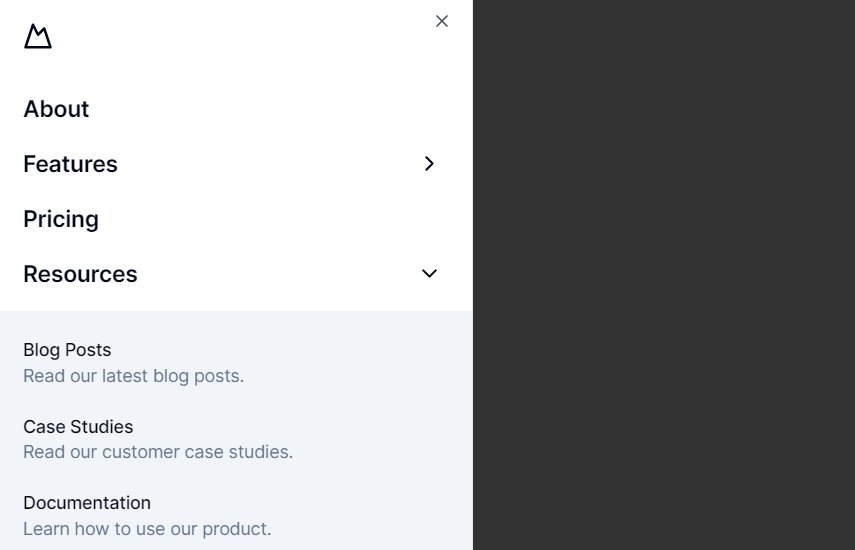
You can See https://v0.dev/aaronnfs