Toggle buttons have become an essential component of modern web interfaces, allowing users to switch between two states with a single click. Bootstrap 5 provides robust tools for creating attractive and functional toggle buttons. This guide explores various implementation approaches and best practices.
Understanding Bootstrap Toggle Buttons
Toggle buttons in Bootstrap 5 maintain a pressed or active state upon activation. This functionality makes them ideal for features that can be turned on or off, such as settings, filters, or display preferences.
Basic Implementation
The foundation of Bootstrap toggle buttons relies on the data-bs-toggle="button"
attribute. Here’s a simple implementation:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Basic Toggle Buttons</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body class="p-4">
<div class="container">
<h5>Basic Toggle Buttons</h5>
<!-- Single Toggle -->
<button type="button" class="btn btn-primary mb-3" data-bs-toggle="button">
Toggle Button
</button>
<!-- Different States -->
<div class="d-flex gap-2 mb-4">
<button type="button" class="btn btn-success" data-bs-toggle="button">
Active Toggle
</button>
<button type="button" class="btn btn-warning" data-bs-toggle="button">
Pressed Toggle
</button>
<button type="button" class="btn btn-danger" disabled data-bs-toggle="button">
Disabled Toggle
</button>
</div>
<!-- Different Sizes -->
<div class="d-flex gap-2 align-items-center">
<button type="button" class="btn btn-primary btn-lg" data-bs-toggle="button">
Large Toggle
</button>
<button type="button" class="btn btn-primary" data-bs-toggle="button">
Default Toggle
</button>
<button type="button" class="btn btn-primary btn-sm" data-bs-toggle="button">
Small Toggle
</button>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
Custom Toggle Switches
For a more modern appearance, you can create custom toggle switches that resemble iOS-style controls:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Custom Toggle Switches</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0/css/all.min.css">
<style>
.toggle-switch {
width: 60px;
height: 30px;
position: relative;
display: inline-block;
}
.toggle-switch input {
opacity: 0;
width: 0;
height: 0;
}
.toggle-slider {
position: absolute;
cursor: pointer;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: #ccc;
transition: .4s;
border-radius: 34px;
}
.toggle-slider:before {
position: absolute;
content: "";
height: 22px;
width: 22px;
left: 4px;
bottom: 4px;
background-color: white;
transition: .4s;
border-radius: 50%;
}
input:checked + .toggle-slider {
background-color: #0d6efd;
}
input:checked + .toggle-slider:before {
transform: translateX(30px);
}
</style>
</head>
<body class="p-4">
<div class="container">
<h5 class="mb-4">Settings</h5>
<!-- Custom Toggle Switches -->
<div class="list-group">
<div class="list-group-item d-flex justify-content-between align-items-center">
<div>
<h6 class="mb-0">Dark Mode</h6>
<small class="text-muted">Enable dark theme</small>
</div>
<label class="toggle-switch mb-0">
<input type="checkbox">
<span class="toggle-slider"></span>
</label>
</div>
<div class="list-group-item d-flex justify-content-between align-items-center">
<div>
<h6 class="mb-0">Notifications</h6>
<small class="text-muted">Enable push notifications</small>
</div>
<label class="toggle-switch mb-0">
<input type="checkbox" checked>
<span class="toggle-slider"></span>
</label>
</div>
<div class="list-group-item d-flex justify-content-between align-items-center">
<div>
<h6 class="mb-0">Auto-Update</h6>
<small class="text-muted">Update automatically</small>
</div>
<label class="toggle-switch mb-0">
<input type="checkbox">
<span class="toggle-slider"></span>
</label>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>

Advanced Implementation Techniques
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Advanced Toggle Buttons</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.0.0/css/all.min.css">
</head>
<body class="p-4">
<div class="container">
<h5 class="mb-4">Feature Controls</h5>
<!-- Icon Toggle Buttons -->
<div class="d-flex gap-3 mb-4">
<button type="button" class="btn btn-outline-primary position-relative" data-bs-toggle="button">
<i class="fas fa-wifi me-2"></i>WiFi
<span class="position-absolute top-0 start-100 translate-middle badge rounded-pill bg-danger">
Off
</span>
</button>
<button type="button" class="btn btn-outline-success position-relative" data-bs-toggle="button">
<i class="fas fa-bluetooth-b me-2"></i>Bluetooth
<span class="position-absolute top-0 start-100 translate-middle badge rounded-pill bg-danger">
Off
</span>
</button>
<button type="button" class="btn btn-outline-info position-relative" data-bs-toggle="button">
<i class="fas fa-plane me-2"></i>Airplane Mode
<span class="position-absolute top-0 start-100 translate-middle badge rounded-pill bg-danger">
Off
</span>
</button>
</div>
<!-- Toggle Button Group -->
<div class="btn-group mb-4" role="group" aria-label="Audio Controls">
<input type="checkbox" class="btn-check" id="microphone" autocomplete="off">
<label class="btn btn-outline-dark" for="microphone">
<i class="fas fa-microphone"></i>
</label>
<input type="checkbox" class="btn-check" id="headphones" autocomplete="off">
<label class="btn btn-outline-dark" for="headphones">
<i class="fas fa-headphones"></i>
</label>
<input type="checkbox" class="btn-check" id="volume" autocomplete="off">
<label class="btn btn-outline-dark" for="volume">
<i class="fas fa-volume-up"></i>
</label>
</div>
<!-- Animated Toggle with Status -->
<div class="card">
<div class="card-body">
<div class="d-flex justify-content-between align-items-center">
<div>
<h6 class="card-title mb-0">Power Saving Mode</h6>
<p class="card-text text-muted mb-0">Conserve battery life</p>
</div>
<button class="btn btn-lg btn-outline-warning rounded-circle" data-bs-toggle="button">
<i class="fas fa-power-off"></i>
</button>
</div>
</div>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
<script>
// Update badge text based on toggle state
document.querySelectorAll('[data-bs-toggle="button"]').forEach(button => {
button.addEventListener('click', function() {
const badge = this.querySelector('.badge');
if (badge) {
badge.textContent = this.classList.contains('active') ? 'On' : 'Off';
badge.classList.toggle('bg-success');
badge.classList.toggle('bg-danger');
}
});
});
</script>
</body>
</html>
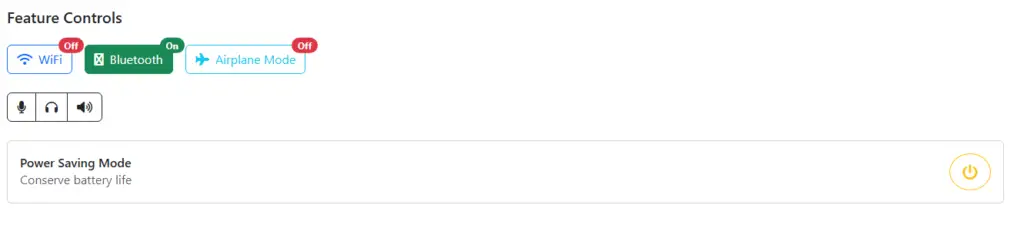