The Bootstrap 4 DateRangePicker offers a robust solution for handling date selection in your web applications. This guide will walk you through implementing this powerful tool with practical examples.
Introduction to Bootstrap DateRangePicker
The DateRangePicker is a JavaScript component that creates an intuitive interface for selecting single dates or date ranges. It integrates seamlessly with Bootstrap 4 and provides a variety of customization options to suit different use cases.
Key Features
- Single date or date range selection
- Time picker functionality
- Predefined date ranges
- Customizable formatting
- Responsive design
- Extensive configuration options
Prerequisites
Before implementing the DateRangePicker, ensure you have included all necessary dependencies:
<!-- CSS Dependencies -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/4.6.0/css/bootstrap.min.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-daterangepicker/3.0.5/daterangepicker.css">
<!-- JavaScript Dependencies -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.1/moment.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-daterangepicker/3.0.5/daterangepicker.min.js"></script>
Implementation Examples
Let’s explore three common implementation scenarios for the DateRangePicker.
1. Basic Date Range Selection
The basic implementation provides a clean interface for selecting a date range. Here’s how to create a simple date range picker:
<div class="form-group">
<label>Basic Date Range Picker</label>
<input type="text" class="form-control" id="basic-picker">
</div>
<script>
$('#basic-picker').daterangepicker({
startDate: moment().subtract(7, 'days'),
endDate: moment(),
opens: 'left',
drops: 'auto',
locale: {
format: 'MM/DD/YYYY'
}
}, function(start, end) {
console.log('Selected date range:', start.format('YYYY-MM-DD'), 'to', end.format('YYYY-MM-DD'));
});
</script>
This configuration creates a date picker that:
- Defaults to the last 7 days
- Opens to the left of the input
- Automatically adjusts its position based on available space
- Uses a standard MM/DD/YYYY format
2. Advanced Range Selection with Presets
For more complex use cases, you might want to include predefined date ranges:
<div class="form-group">
<label>Date Range Picker with Predefined Ranges</label>
<input type="text" class="form-control" id="ranges-picker">
</div>
<script>
$('#ranges-picker').daterangepicker({
startDate: moment().startOf('month'),
endDate: moment().endOf('month'),
ranges: {
'Today': [moment(), moment()],
'Yesterday': [moment().subtract(1, 'days'), moment().subtract(1, 'days')],
'Last 7 Days': [moment().subtract(6, 'days'), moment()],
'Last 30 Days': [moment().subtract(29, 'days'), moment()],
'This Month': [moment().startOf('month'), moment().endOf('month')],
'Last Month': [moment().subtract(1, 'month').startOf('month'),
moment().subtract(1, 'month').endOf('month')]
},
locale: {
format: 'MM/DD/YYYY'
}
}, function(start, end, label) {
console.log('Selected range:', label);
});
</script>
This implementation adds convenient preset ranges that users can select with a single click, improving efficiency for common date range selections.
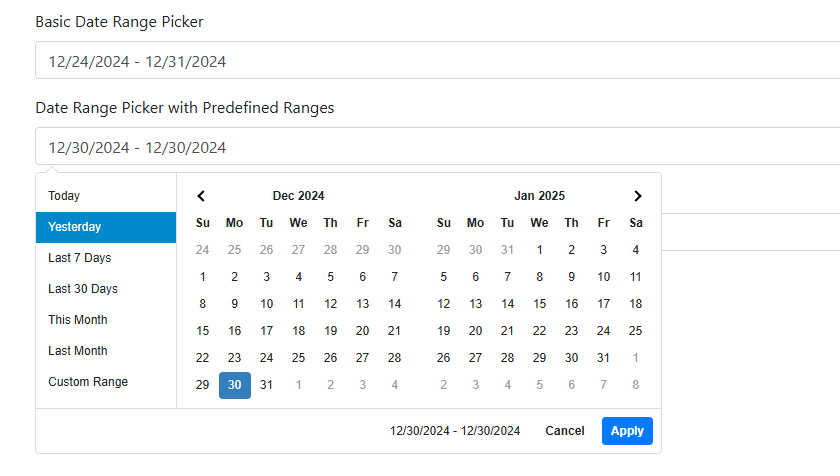
3. Single Date Selection with Time
For scenarios requiring precise datetime selection:
<div class="form-group">
<label>Single Date Picker with Time</label>
<input type="text" class="form-control" id="single-picker">
</div>
<script>
$('#single-picker').daterangepicker({
singleDatePicker: true,
timePicker: true,
timePicker24Hour: false,
timePickerIncrement: 15,
locale: {
format: 'MM/DD/YYYY hh:mm A'
}
}, function(start) {
console.log('Selected datetime:', start.format('YYYY-MM-DD HH:mm:ss'));
});
</script>
This configuration creates a single date picker with time selection capabilities, perfect for scheduling applications or event management systems.
Best Practices and Tips
When implementing the DateRangePicker, consider the following recommendations:
- Input Format Consistency Maintain consistent date formats throughout your application. The
locale.format
option helps ensure uniformity in how dates are displayed. - Position Consideration Use the
opens
anddrops
options to control where the picker appears. This is particularly important for mobile responsiveness and user experience. - Event Handling Implement appropriate callback functions to handle date selection events. This allows you to perform necessary actions when users select dates.
- Validation Add date validation logic within the callback function to ensure selected dates meet your application’s requirements.
Common Customization Options
The DateRangePicker offers numerous customization options:
{
startDate: moment().startOf('day'),
endDate: moment().endOf('day'),
minDate: moment().subtract(1, 'years'),
maxDate: moment().add(1, 'years'),
timePicker: true,
timePickerIncrement: 30,
locale: {
format: 'MM/DD/YYYY',
separator: ' - ',
applyLabel: 'Apply',
cancelLabel: 'Cancel',
fromLabel: 'From',
toLabel: 'To',
customRangeLabel: 'Custom Range'
},
opens: 'left',
drops: 'down',
showDropdowns: true,
showWeekNumbers: true,
showISOWeekNumbers: true,
showCustomRangeLabel: true,
alwaysShowCalendars: true,
autoUpdateInput: true,
linkedCalendars: true
}
Conclusion
The Bootstrap 4 DateRangePicker is a versatile tool that can significantly enhance the user experience of your web applications. By understanding its configuration options and implementation patterns, you can create intuitive date selection interfaces that meet your specific requirements.