In this tutorial, we will create autocomplete in react with ant design 5. First you need to setup react with ant design 5 project.
install & setup vite + react + typescript + ant design 5
1. Create a simple autocomplete in React using Ant Design 5’s AutoComplete component and the useState hook.
import React, { useState } from 'react';
import { AutoComplete } from 'antd';
const mockVal = (str: string, repeat = 1) => ({
value: str.repeat(repeat),
});
const App: React.FC = () => {
const [value, setValue] = useState('');
const [options, setOptions] = useState<{ value: string }[]>([]);
const [anotherOptions, setAnotherOptions] = useState<{ value: string }[]>([]);
const getPanelValue = (searchText: string) =>
!searchText ? [] : [mockVal(searchText), mockVal(searchText, 2), mockVal(searchText, 3)];
const onSelect = (data: string) => {
console.log('onSelect', data);
};
const onChange = (data: string) => {
setValue(data);
};
return (
<>
<AutoComplete
options={options}
style={{ width: 200 }}
onSelect={onSelect}
onSearch={(text) => setOptions(getPanelValue(text))}
placeholder="input here"
/>
<br />
<br />
<AutoComplete
value={value}
options={anotherOptions}
style={{ width: 200 }}
onSelect={onSelect}
onSearch={(text) => setAnotherOptions(getPanelValue(text))}
onChange={onChange}
placeholder="control mode"
/>
</>
);
};
export default App;
2. react ant design 5 search autocomplete with options property.
import React from 'react';
import { UserOutlined } from '@ant-design/icons';
import { AutoComplete, Input } from 'antd';
const renderTitle = (title: string) => (
<span>
{title}
<a
style={{ float: 'right' }}
href="https://www.google.com/search?q=antd"
target="_blank"
rel="noopener noreferrer"
>
more
</a>
</span>
);
const renderItem = (title: string, count: number) => ({
value: title,
label: (
<div
style={{
display: 'flex',
justifyContent: 'space-between',
}}
>
{title}
<span>
<UserOutlined /> {count}
</span>
</div>
),
});
const options = [
{
label: renderTitle('Libraries'),
options: [renderItem('AntDesign', 10000), renderItem('AntDesign UI', 10600)],
},
{
label: renderTitle('Solutions'),
options: [renderItem('AntDesign UI FAQ', 60100), renderItem('AntDesign FAQ', 30010)],
},
{
label: renderTitle('Articles'),
options: [renderItem('AntDesign design language', 100000)],
},
];
const App: React.FC = () => (
<AutoComplete
popupClassName="certain-category-search-dropdown"
dropdownMatchSelectWidth={500}
style={{ width: 250 }}
options={options}
>
<Input.Search size="large" placeholder="input here" />
</AutoComplete>
);
export default App;
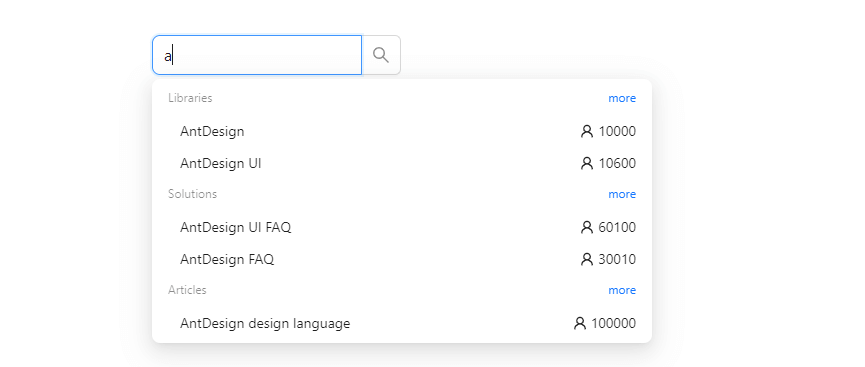
3. react typescript with ant design 5 search autocomplete.
import React, { useState } from 'react';
import { AutoComplete, Input } from 'antd';
import type { SelectProps } from 'antd/es/select';
const getRandomInt = (max: number, min = 0) => Math.floor(Math.random() * (max - min + 1)) + min;
const searchResult = (query: string) =>
new Array(getRandomInt(5))
.join('.')
.split('.')
.map((_, idx) => {
const category = `${query}${idx}`;
return {
value: category,
label: (
<div
style={{
display: 'flex',
justifyContent: 'space-between',
}}
>
<span>
Found {query} on{' '}
<a
href={`https://s.taobao.com/search?q=${query}`}
target="_blank"
rel="noopener noreferrer"
>
{category}
</a>
</span>
<span>{getRandomInt(200, 100)} results</span>
</div>
),
};
});
const App: React.FC = () => {
const [options, setOptions] = useState<SelectProps<object>['options']>([]);
const handleSearch = (value: string) => {
setOptions(value ? searchResult(value) : []);
};
const onSelect = (value: string) => {
console.log('onSelect', value);
};
return (
<AutoComplete
dropdownMatchSelectWidth={252}
style={{ width: 300 }}
options={options}
onSelect={onSelect}
onSearch={handleSearch}
>
<Input.Search size="large" placeholder="input here" enterButton />
</AutoComplete>
);
};
export default App;
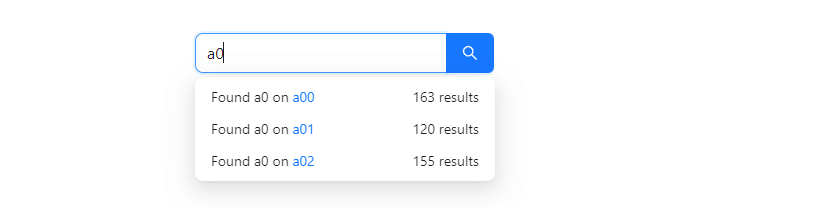