In this tutorial, we’ll create carousel slider in React Material UI 5.
Install & Setup Vite + React + Typescript + MUI 5
To use a carousel slider in React MUI 5, you need to install react-material-ui-carousel.
npm install react-material-ui-carousel --save
Note: You will need to have Material UI installed, in order to use this library/component.
npm install @mui/material
npm install @mui/icons-material
npm install @mui/styles
We use Carousel from react-material-ui-carousel and style it with Paper. Item renders slide content, and we add a Learn More button for interaction.
import React from "react";
import { Paper, Box, Typography, Button } from "@mui/material";
import Carousel from "react-material-ui-carousel";
const exampleItems = [
{
name: "First Item",
description: "Description for first item",
},
{
name: "Second Item",
description: "Description for second item",
},
];
const ExampleCarousel = () => {
return (
<Carousel
animation="slide"
indicators={true}
timeout={500}
navButtonsAlwaysVisible={true}
navButtonsAlwaysInvisible={false}
cycleNavigation={true}
fullHeightHover={false}
sx={{
maxWidth: "600px",
flexGrow: 1,
margin: "auto",
mt: 5,
}}
>
{exampleItems.map((item, i) => (
<Item key={i} item={item} />
))}
</Carousel>
);
};
function Item(props) {
return (
<Paper
sx={{
position: "relative",
backgroundColor: "grey.100",
color: "#fff",
backgroundSize: "cover",
backgroundRepeat: "no-repeat",
backgroundPosition: "center",
justifyContent: "center",
alignItems: "center",
height: "300px",
display: "flex",
flexDirection: "column",
borderRadius: "10px",
p: 4,
}}
elevation={10}
>
<Typography variant="h4">{props.item.name}</Typography>
<Typography>{props.item.description}</Typography>
<Button
variant="contained"
color="primary"
sx={{ mt: 2, alignSelf: "center" }}
>
Learn More
</Button>
</Paper>
);
}
export default ExampleCarousel;
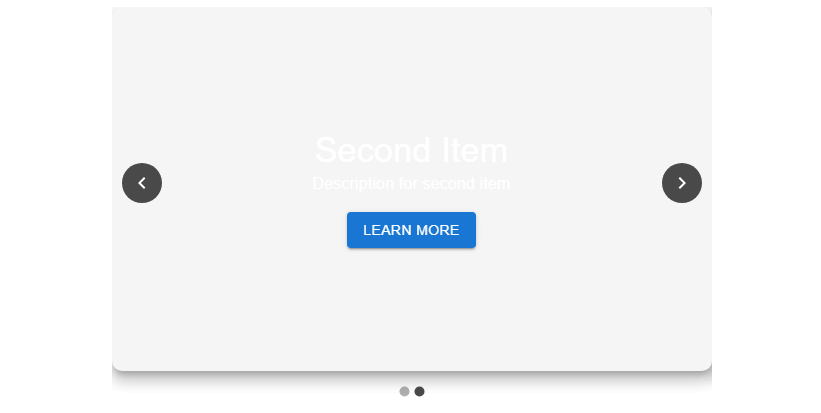
React Material UI 5 Image Gallery Carousel
Create a basic image gallery carousel slider with react-material-ui-carousel and react mui 5.
import React from 'react';
import Carousel from 'react-material-ui-carousel';
import { Paper, Box } from '@mui/material';
const images = [
"https://cdn.pixabay.com/photo/2023/10/02/14/51/flowers-8289321_640.png",
"https://cdn.pixabay.com/photo/2023/09/10/15/15/flowers-8245210_640.png",
"https://cdn.pixabay.com/photo/2023/09/04/17/04/saturn-8233220_640.png"
];
function ImageCarousel() {
return (
<Box sx={{ maxWidth: 800, flexGrow: 1, margin: 'auto', mt: 5 }}>
<Carousel>
{images.map((image, i) => (
<Paper key={i} elevation={10}>
<Box
component="img"
sx={{
width: '100%',
height: '500px',
objectFit: 'cover'
}}
src={image}
alt={`Slide ${i}`}
/>
</Paper>
))}
</Carousel>
</Box>
);
}
export default ImageCarousel;
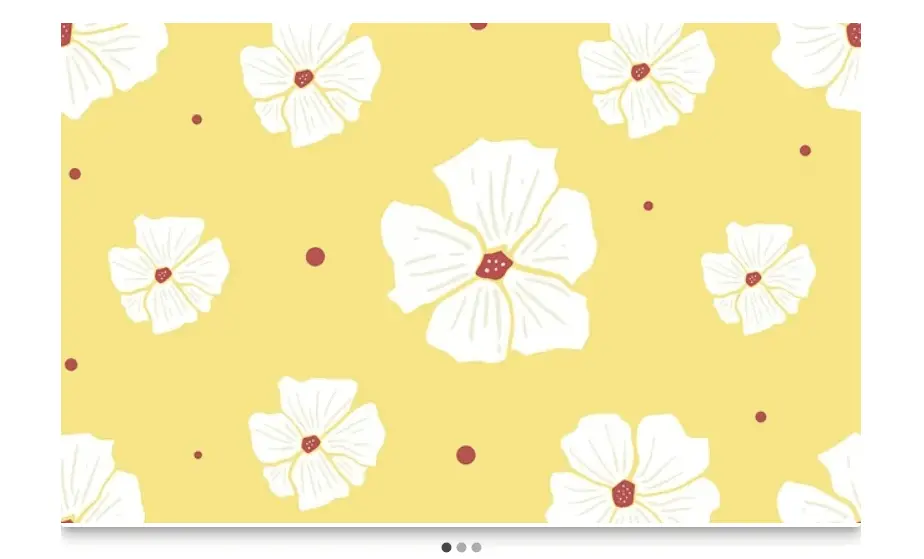
React Material UI 5 Testimonials Carousel
We will create a React MUI 5 carousel that displays customer testimonials.
import React from 'react';
import Carousel from 'react-material-ui-carousel';
import { Paper, Typography, Avatar, Box } from '@mui/material';
const testimonials = [
{
avatar: "/user1.jpg",
name: "John Doe",
text: "The service was amazing and the staff was very friendly!"
},
{
avatar: "/user2.jpg",
name: "Jane Smith",
text: "I had a great experience, and I would definitely come back again!"
}
];
function TestimonialCarousel() {
return (
<Carousel autoPlay={false} animation="slide">
{testimonials.map((testimonial, i) => (
<Paper key={i} elevation={10} sx={{ padding: 4, margin: 'auto', maxWidth: 800 }}>
<Box sx={{ display: 'flex', alignItems: 'center', flexDirection: 'column' }}>
<Avatar src={testimonial.avatar} alt={testimonial.name} sx={{ width: 90, height: 90, mb: 2 }} />
<Typography variant="subtitle1" component="h3" sx={{ fontStyle: 'italic' }}>
"{testimonial.text}"
</Typography>
<Typography variant="body2" component="p" sx={{ mt: 1 }}>
- {testimonial.name}
</Typography>
</Box>
</Paper>
))}
</Carousel>
);
}
export default TestimonialCarousel;
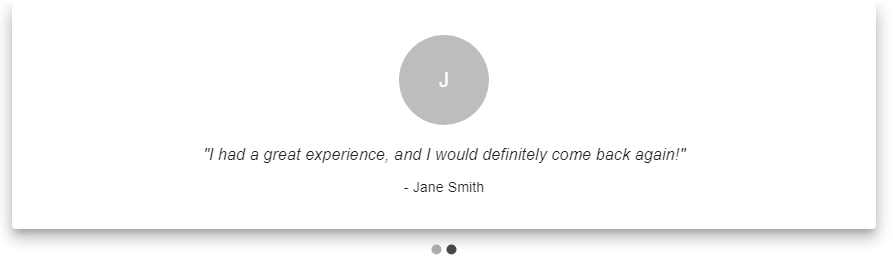