In this tutorial, we’ll delve into implementing Notifications in React using Ant Design. We’ll explore how to utilize Ant Design 5 Notifications with TypeScript, showcasing examples of notifications positioned at the left, right, top, and bottom.
install & setup vite + react + typescript + ant design 5
1. Utilize React with Ant Design 5 and TypeScript to showcase Notifications positioned at the left, right, top, and bottom.
import React, { useMemo } from 'react';
import {
RadiusBottomleftOutlined,
RadiusBottomrightOutlined,
RadiusUpleftOutlined,
RadiusUprightOutlined,
} from '@ant-design/icons';
import { Button, Divider, notification, Space } from 'antd';
import type { NotificationArgsProps } from 'antd';
type NotificationPlacement = NotificationArgsProps['placement'];
const Context = React.createContext({ name: 'Default' });
const App: React.FC = () => {
const [api, contextHolder] = notification.useNotification();
const openNotification = (placement: NotificationPlacement) => {
api.info({
message: `Notification ${placement}`,
description: <Context.Consumer>{({ name }) => `Hello, ${name}!`}</Context.Consumer>,
placement,
});
};
const contextValue = useMemo(() => ({ name: 'Ant Design' }), []);
return (
<Context.Provider value={contextValue}>
{contextHolder}
<Space>
<Button
type="primary"
onClick={() => openNotification('topLeft')}
icon={<RadiusUpleftOutlined />}
>
topLeft
</Button>
<Button
type="primary"
onClick={() => openNotification('topRight')}
icon={<RadiusUprightOutlined />}
>
topRight
</Button>
</Space>
<Divider />
<Space>
<Button
type="primary"
onClick={() => openNotification('bottomLeft')}
icon={<RadiusBottomleftOutlined />}
>
bottomLeft
</Button>
<Button
type="primary"
onClick={() => openNotification('bottomRight')}
icon={<RadiusBottomrightOutlined />}
>
bottomRight
</Button>
</Space>
</Context.Provider>
);
};
export default App;
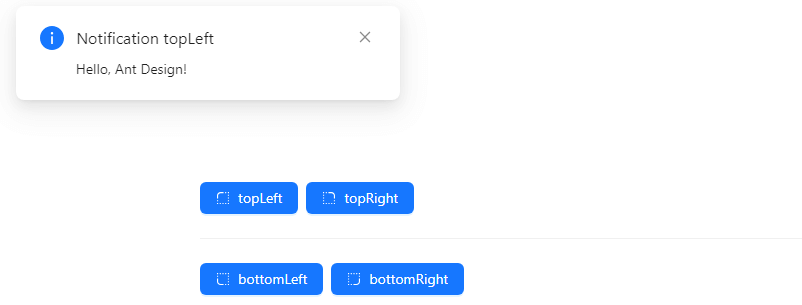
2. React with Ant Design Open the notification box with Custom close button.
import React from 'react';
import { Button, notification, Space } from 'antd';
const close = () => {
console.log(
'Notification was closed. Either the close button was clicked or duration time elapsed.',
);
};
const App: React.FC = () => {
const [api, contextHolder] = notification.useNotification();
const openNotification = () => {
const key = `open${Date.now()}`;
const btn = (
<Space>
<Button type="link" size="small" onClick={() => api.destroy()}>
Destroy All
</Button>
<Button type="primary" size="small" onClick={() => api.destroy(key)}>
Confirm
</Button>
</Space>
);
api.open({
message: 'Notification Title',
description:
'A function will be be called after the notification is closed (automatically after the "duration" time of manually).',
btn,
key,
onClose: close,
});
};
return (
<>
{contextHolder}
<Button type="primary" onClick={openNotification}>
Open the notification box
</Button>
</>
);
};
export default App;

3. Build a React app with TypeScript and Ant Design, including Notifications for success, info, warning, and error messages.
import React from 'react';
import { Button, notification, Space } from 'antd';
type NotificationType = 'success' | 'info' | 'warning' | 'error';
const App: React.FC = () => {
const [api, contextHolder] = notification.useNotification();
const openNotificationWithIcon = (type: NotificationType) => {
api[type]({
message: 'Notification Title',
description:
'This is the content of the notification. This is the content of the notification. This is the content of the notification.',
});
};
return (
<>
{contextHolder}
<Space>
<Button onClick={() => openNotificationWithIcon('success')}>Success</Button>
<Button onClick={() => openNotificationWithIcon('info')}>Info</Button>
<Button onClick={() => openNotificationWithIcon('warning')}>Warning</Button>
<Button onClick={() => openNotificationWithIcon('error')}>Error</Button>
</Space>
</>
);
};
export default App;
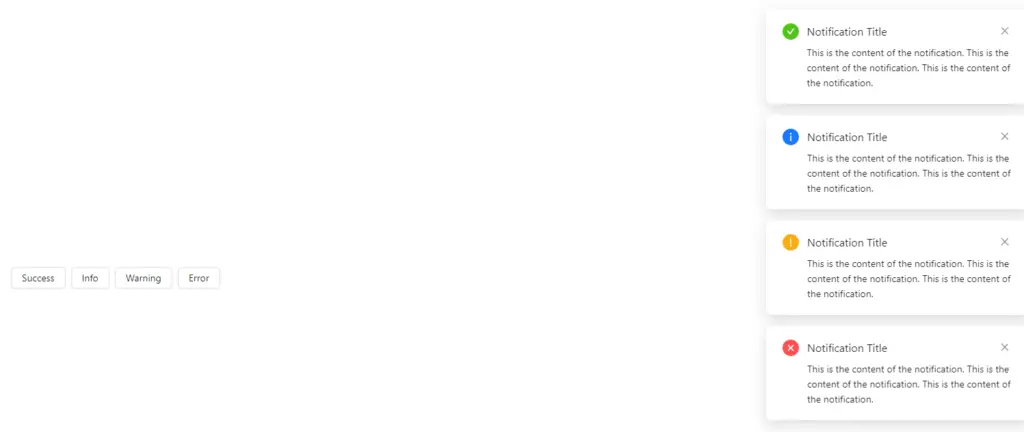