Learn how to create a floating button in React using Ant Design 5. This tutorial assumes you have already set up a React project with Ant Design 5, a powerful UI framework known for its sleek components and ease of use. Follow along to add a stylish floating button to your React application effortlessly.
install & setup vite + react + typescript + ant design 5
1. Create a simple floating button using React and Ant Design 5 with the FloatButton
component from react-antd
.
import React from 'react';
import { FloatButton } from 'antd';
const App: React.FC = () => <FloatButton onClick={() => console.log('click')} />;
export default App;
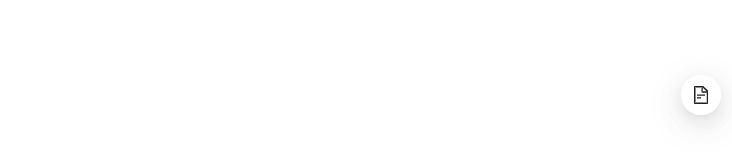
2. React ant design 5 floating button with type.
import React from 'react';
import { QuestionCircleOutlined } from '@ant-design/icons';
import { FloatButton } from 'antd';
const App: React.FC = () => (
<>
<FloatButton icon={<QuestionCircleOutlined />} type="primary" style={{ right: 24 }} />
<FloatButton icon={<QuestionCircleOutlined />} type="default" style={{ right: 94 }} />
</>
);
export default App;
3. React ant design 5 floating button with icon.
import React from 'react';
import { CustomerServiceOutlined } from '@ant-design/icons';
import { FloatButton } from 'antd';
const App: React.FC = () => (
<>
<FloatButton
shape="circle"
type="primary"
style={{ right: 94 }}
icon={<CustomerServiceOutlined />}
/>
<FloatButton
shape="square"
type="primary"
style={{ right: 24 }}
icon={<CustomerServiceOutlined />}
/>
</>
);
export default App;
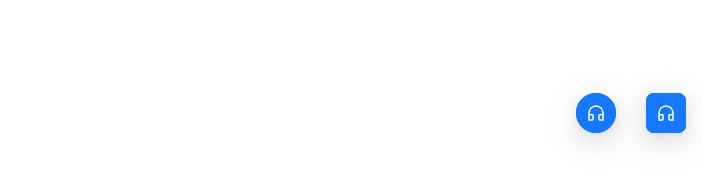
4. react ant design 5 floating button with description.
import React from 'react';
import { FileTextOutlined } from '@ant-design/icons';
import { FloatButton } from 'antd';
const App: React.FC = () => (
<>
<FloatButton
icon={<FileTextOutlined />}
description="HELP INFO"
shape="square"
style={{ right: 24 }}
/>
<FloatButton description="HELP INFO" shape="square" style={{ right: 94 }} />
<FloatButton
icon={<FileTextOutlined />}
description="HELP"
shape="square"
style={{ right: 164 }}
/>
</>
);
export default App;
5. React ant design 5 floating button with tooltip.
import React from 'react';
import { FloatButton } from 'antd';
const App: React.FC = () => <FloatButton tooltip={<div>Documents</div>} />;
export default App;
6. React ant design 5 floating button with group.
import React from 'react';
import { QuestionCircleOutlined, SyncOutlined } from '@ant-design/icons';
import { FloatButton } from 'antd';
const App: React.FC = () => (
<>
<FloatButton.Group shape="circle" style={{ right: 24 }}>
<FloatButton icon={<QuestionCircleOutlined />} />
<FloatButton />
<FloatButton.BackTop visibilityHeight={0} />
</FloatButton.Group>
<FloatButton.Group shape="square" style={{ right: 94 }}>
<FloatButton icon={<QuestionCircleOutlined />} />
<FloatButton />
<FloatButton icon={<SyncOutlined />} />
<FloatButton.BackTop visibilityHeight={0} />
</FloatButton.Group>
</>
);
export default App;
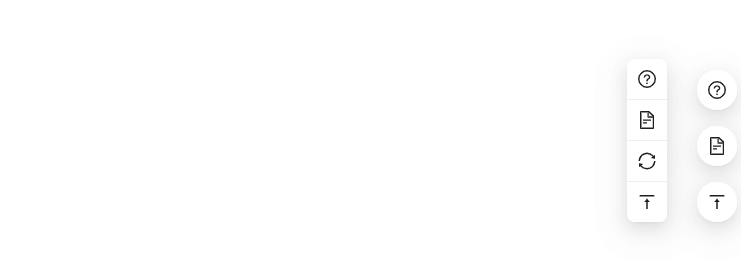
7. React ant design 5 floating button open menu mode with trigger, which could be hover or click.
import { CommentOutlined, CustomerServiceOutlined } from '@ant-design/icons';
import React from 'react';
import { FloatButton } from 'antd';
const App: React.FC = () => (
<>
<FloatButton.Group
trigger="click"
type="primary"
style={{ right: 24 }}
icon={<CustomerServiceOutlined />}
>
<FloatButton />
<FloatButton icon={<CommentOutlined />} />
</FloatButton.Group>
<FloatButton.Group
trigger="hover"
type="primary"
style={{ right: 94 }}
icon={<CustomerServiceOutlined />}
>
<FloatButton />
<FloatButton icon={<CommentOutlined />} />
</FloatButton.Group>
</>
);
export default App;
8. React ant design 5 floating button set the component to controlled mode through open, which need to be used together with trigger.
import { CommentOutlined, CustomerServiceOutlined } from '@ant-design/icons';
import React, { useState } from 'react';
import { FloatButton, Switch } from 'antd';
const App: React.FC = () => {
const [open, setOpen] = useState(true);
const onChange = (checked: boolean) => {
setOpen(checked);
};
return (
<>
<FloatButton.Group
open={open}
trigger="click"
style={{ right: 24 }}
icon={<CustomerServiceOutlined />}
>
<FloatButton />
<FloatButton icon={<CommentOutlined />} />
</FloatButton.Group>
<Switch onChange={onChange} checked={open} style={{ margin: 16 }} />
</>
);
};
export default App;
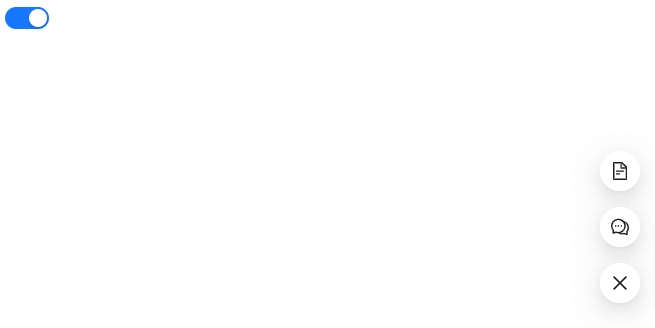
9. React ant design 5 floating button with back top button.
import React from 'react';
import { FloatButton } from 'antd';
const App: React.FC = () => (
<div style={{ height: '500vh', padding: 10 }}>
<div>Scroll to bottom</div>
<div>Scroll to bottom</div>
<div>Scroll to bottom</div>
<div>Scroll to bottom</div>
<div>Scroll to bottom</div>
<div>Scroll to bottom</div>
<div>Scroll to bottom</div>
<FloatButton.BackTop />
</div>
);
export default App;
10. React ant design 5 floating button with badge with icon notification.
import { QuestionCircleOutlined } from '@ant-design/icons';
import React from 'react';
import { FloatButton } from 'antd';
const App: React.FC = () => (
<>
<FloatButton shape="circle" badge={{ dot: true }} style={{ right: 24 + 70 + 70 }} />
<FloatButton.Group shape="circle" style={{ right: 24 + 70 }}>
<FloatButton tooltip={<div>custom badge color</div>} badge={{ count: 5, color: 'blue' }} />
<FloatButton badge={{ count: 5 }} />
</FloatButton.Group>
<FloatButton.Group shape="circle">
<FloatButton badge={{ count: 12 }} icon={<QuestionCircleOutlined />} />
<FloatButton badge={{ count: 123, overflowCount: 999 }} />
<FloatButton.BackTop visibilityHeight={0} />
</FloatButton.Group>
</>
);
export default App;
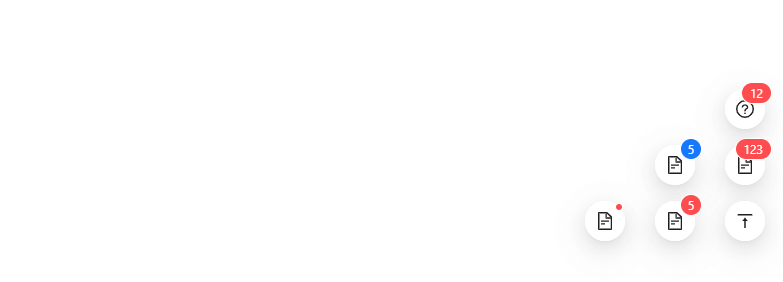