In this tutorial, we will create a login form page in React using Ant Design 5. We will demonstrate how to build a login form with TypeScript and showcase a sign-in form with simple validation examples using React and Ant Design 5.
install & setup vite + react + typescript + ant design 5
React Ant Design 5 Login Form Example
1. Create a login form in React using Ant Design 5 components: Button
, Checkbox
, Form
, and Input
.
import React from 'react';
import { Button, Checkbox, Form, Input } from 'antd';
const onFinish = (values: any) => {
console.log('Success:', values);
};
const onFinishFailed = (errorInfo: any) => {
console.log('Failed:', errorInfo);
};
const App: React.FC = () => (
<Form
name="basic"
labelCol={{ span: 8 }}
wrapperCol={{ span: 16 }}
style={{ maxWidth: 600 }}
initialValues={{ remember: true }}
onFinish={onFinish}
onFinishFailed={onFinishFailed}
autoComplete="off"
>
<Form.Item
label="Username"
name="username"
rules={[{ required: true, message: 'Please input your username!' }]}
>
<Input />
</Form.Item>
<Form.Item
label="Password"
name="password"
rules={[{ required: true, message: 'Please input your password!' }]}
>
<Input.Password />
</Form.Item>
<Form.Item name="remember" valuePropName="checked" wrapperCol={{ offset: 8, span: 16 }}>
<Checkbox>Remember me</Checkbox>
</Form.Item>
<Form.Item wrapperCol={{ offset: 8, span: 16 }}>
<Button type="primary" htmlType="submit">
Submit
</Button>
</Form.Item>
</Form>
);
export default App;
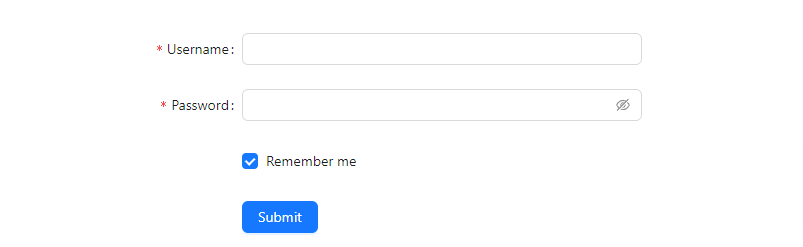
2. Create a login form in React using Ant Design 5 Card
with a “Forgot Password” option and icons inside the input fields.
import React from "react";
import { Form, Input, Button, Checkbox, Card } from "antd";
import { UserOutlined, LockOutlined } from "@ant-design/icons";
import { Typography } from "antd";
const { Title } = Typography;
const LoginForm = () => {
const onFinish = (values) => {
console.log("Received values of form: ", values);
if (values.remember) {
localStorage.setItem("username", values.username);
localStorage.setItem("password", values.password);
}
};
const handleForgotPassword = (e) => {
e.preventDefault();
console.log("Handle password recovery logic here");
};
const handleRegister = (e) => {
e.preventDefault();
console.log("Handle registration logic here");
};
return (
<div
style={{
display: "flex",
justifyContent: "center",
alignItems: "center",
height: "100vh",
}}
>
<Card style={{ width: 500 }}>
<div style={{ display: "flex", justifyContent: "center" }}>
<Title level={2}>Company Logo </Title>
</div>
<Form
name="normal_login"
className="login-form"
initialValues={{ remember: true }}
onFinish={onFinish}
>
<Form.Item
name="username"
rules={[{ required: true, message: "Please input your Username!" }]}
>
<Input
prefix={<UserOutlined className="site-form-item-icon" />}
placeholder="Username"
/>
</Form.Item>
<Form.Item
name="password"
rules={[{ required: true, message: "Please input your Password!" }]}
>
<Input
prefix={<LockOutlined className="site-form-item-icon" />}
type="password"
placeholder="Password"
/>
<a
style={{ float: "right" }}
className="login-form-forgot"
href=""
onClick={handleForgotPassword}
>
Forgot password
</a>
</Form.Item>
<Form.Item>
<Form.Item name="remember" valuePropName="checked" noStyle>
<Checkbox>Remember me</Checkbox>
</Form.Item>
</Form.Item>
<Form.Item>
<Button
type="primary"
htmlType="submit"
className="login-form-button"
block
>
Log in
</Button>
Don't have an account{" "}
<a href="" onClick={handleRegister}>
sign up
</a>
</Form.Item>
</Form>
</Card>
</div>
);
};
export default LoginForm;
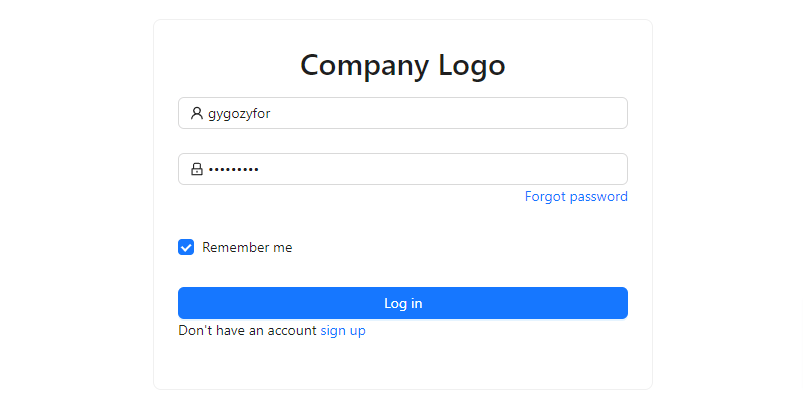
3. Create a login form page in React using Ant Design 5 with simple validation, implemented with TypeScript.
import { FC, FormEvent } from "react";
import { Form, Input, Button, Checkbox, Card, Typography } from "antd";
import { UserOutlined, LockOutlined } from "@ant-design/icons";
const { Title } = Typography;
interface Values {
username: string;
password: string;
remember: boolean;
}
const LoginForm: FC = () => {
const onFinish = (values: Values) => {
console.log("Received values of form: ", values);
if (values.remember) {
localStorage.setItem("username", values.username);
localStorage.setItem("password", values.password);
}
};
const handleForgotPassword = (e: FormEvent) => {
e.preventDefault();
console.log("Handle password recovery logic here");
};
const handleRegister = (e: FormEvent) => {
e.preventDefault();
console.log("Handle registration logic here");
};
return (
<div
style={{
display: "flex",
justifyContent: "center",
alignItems: "center",
height: "100vh",
}}
>
<Card style={{ width: 500 }}>
<div style={{ display: "flex", justifyContent: "center" }}>
<Title level={2}>Company Logo </Title>
</div>
<Form
name="normal_login"
className="login-form"
initialValues={{ remember: true }}
onFinish={onFinish}
>
<Form.Item
name="username"
rules={[{ required: true, message: "Please input your Username!" }]}
>
<Input
prefix={<UserOutlined className="site-form-item-icon" />}
placeholder="Username"
/>
</Form.Item>
<Form.Item
name="password"
rules={[{ required: true, message: "Please input your Password!" }]}
>
<Input
prefix={<LockOutlined className="site-form-item-icon" />}
type="password"
placeholder="Password"
/>
<a
style={{ float: "right" }}
className="login-form-forgot"
href=""
onClick={handleForgotPassword}
>
Forgot password
</a>
</Form.Item>
<Form.Item>
<Form.Item name="remember" valuePropName="checked" noStyle>
<Checkbox>Remember me</Checkbox>
</Form.Item>
</Form.Item>
<Form.Item>
<Button
type="primary"
htmlType="submit"
className="login-form-button"
block
>
Log in
</Button>
Don't have an account{" "}
<a href="" onClick={handleRegister}>
sign up
</a>
</Form.Item>
</Form>
</Card>
</div>
);
};
export default LoginForm;
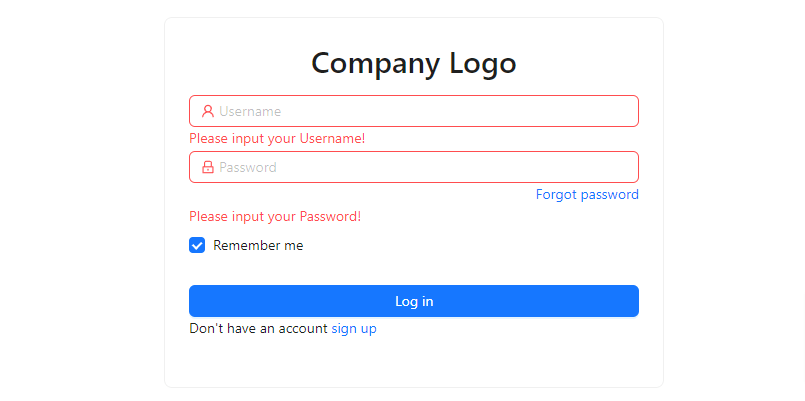