In today’s tutorial, we will create a 404 error page in react js using mui 5 . We will create react material ui 404 not found page, 404 error page with image, we will design 404 page. First you need to install & setup mui 5 in react js.
Install & Setup Vite + React + Typescript + MUI 5
React MUI 5 404 Page Example
1. Simple react mui 5 404 page.
import React from 'react';
import { Box, Typography } from '@mui/material';
import { purple } from '@mui/material/colors';
const primary = purple[500]; // #f44336
export default function Error() {
return (
<Box
sx={{
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
minHeight: '100vh',
backgroundColor: primary,
}}
>
<Typography variant="h1" style={{ color: 'white' }}>
404
</Typography>
</Box>
);
}
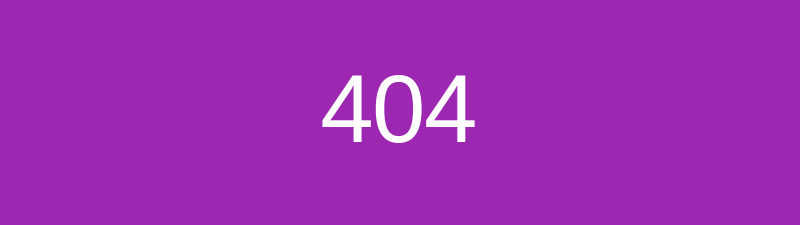
2. React mui 5 404 page not found.
import React from 'react';
import { Box, Button, Typography } from '@mui/material';
import { purple } from '@mui/material/colors';
const primary = purple[500]; // #f44336
export default function Error() {
return (
<Box
sx={{
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
flexDirection: 'column',
minHeight: '100vh',
backgroundColor: primary,
}}
>
<Typography variant="h1" style={{ color: 'white' }}>
404
</Typography>
<Typography variant="h6" style={{ color: 'white' }}>
The page you’re looking for doesn’t exist.
</Typography>
<Button variant="contained">Back Home</Button>
</Box>
);
}
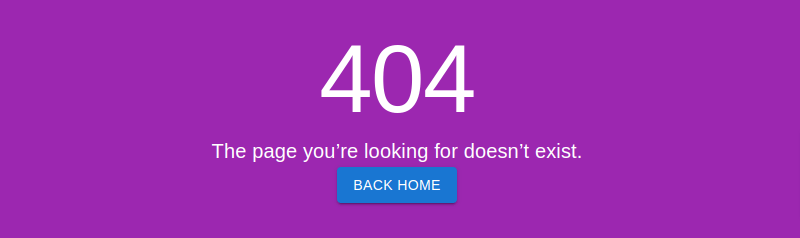
3. React mui 404 page with image.
import React from 'react';
import { Box, Button, Container, Typography } from '@mui/material';
import Grid from '@mui/material/Grid';
export default function Error() {
return (
<Box
sx={{
display: 'flex',
justifyContent: 'center',
alignItems: 'center',
minHeight: '100vh'
}}
>
<Container maxWidth="md">
<Grid container spacing={2}>
<Grid xs={6}>
<Typography variant="h1">
404
</Typography>
<Typography variant="h6">
The page you’re looking for doesn’t exist.
</Typography>
<Button variant="contained">Back Home</Button>
</Grid>
<Grid xs={6}>
<img
src="https://cdn.pixabay.com/photo/2017/03/09/12/31/error-2129569__340.jpg"
alt=""
width={500} height={250}
/>
</Grid>
</Grid>
</Container>
</Box>
);
}
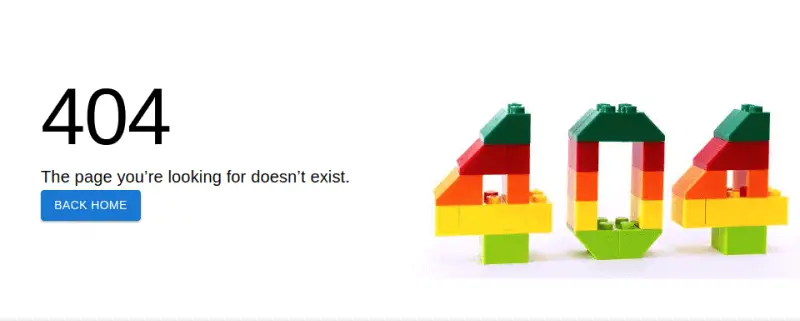