Welcome to our tutorial! We’re here to help you build a login page in React, powered by Material-UI (v5). We’ll walk you through the process of creating a simple and visually appealing sign-in page, complete with images, using React Material UI 5.
Install & Setup Vite + React + Typescript + MUI 5
React Material UI 5 Login Page Example
1. Creating a Simple Login Page with React Material-UI 5.
import Button from "@mui/material/Button";
import TextField from "@mui/material/TextField";
import FormControlLabel from "@mui/material/FormControlLabel";
import Checkbox from "@mui/material/Checkbox";
import Link from "@mui/material/Link";
import Grid from "@mui/material/Grid";
import Box from "@mui/material/Box";
import Typography from "@mui/material/Typography";
import Container from "@mui/material/Container";
export default function SignIn() {
const handleSubmit = (event) => {
event.preventDefault();
const data = new FormData(event.currentTarget);
console.log({
email: data.get("email"),
password: data.get("password"),
});
};
return (
<Container component="main" maxWidth="xs">
<Box
sx={{
marginTop: 8,
display: "flex",
flexDirection: "column",
alignItems: "center",
}}
>
<Typography component="h1" variant="h5">
Sign in
</Typography>
<Box component="form" onSubmit={handleSubmit} noValidate sx={{ mt: 1 }}>
<TextField
margin="normal"
required
fullWidth
id="email"
label="Email Address"
name="email"
autoComplete="email"
autoFocus
/>
<TextField
margin="normal"
required
fullWidth
name="password"
label="Password"
type="password"
id="password"
autoComplete="current-password"
/>
<FormControlLabel
control={<Checkbox value="remember" color="primary" />}
label="Remember me"
/>
<Button
type="submit"
fullWidth
variant="contained"
sx={{ mt: 3, mb: 2 }}
>
Sign In
</Button>
<Grid container>
<Grid item xs>
<Link href="#" variant="body2">
Forgot password?
</Link>
</Grid>
<Grid item>
<Link href="#" variant="body2">
{"Don't have an account? Sign Up"}
</Link>
</Grid>
</Grid>
</Box>
</Box>
</Container>
);
}
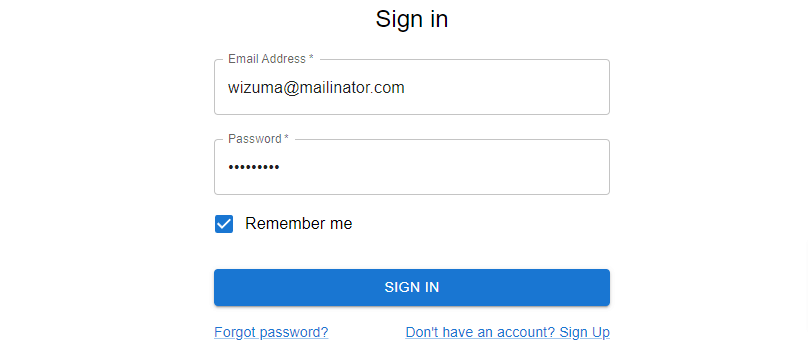
2. Designing a Material-UI 5 Login Page in React with Box Shadow.
import Button from "@mui/material/Button";
import TextField from "@mui/material/TextField";
import FormControlLabel from "@mui/material/FormControlLabel";
import Checkbox from "@mui/material/Checkbox";
import Link from "@mui/material/Link";
import Grid from "@mui/material/Grid";
import Box from "@mui/material/Box";
import Typography from "@mui/material/Typography";
import Container from "@mui/material/Container";
export default function SignIn() {
const handleSubmit = (event) => {
event.preventDefault();
const data = new FormData(event.currentTarget);
console.log({
email: data.get("email"),
password: data.get("password"),
});
};
return (
<Container component="main" maxWidth="sm">
<Box
sx={{
boxShadow: 3,
borderRadius: 2,
px: 4,
py: 6,
marginTop: 8,
display: "flex",
flexDirection: "column",
alignItems: "center",
}}
>
<Typography component="h1" variant="h5">
Sign in
</Typography>
<Box component="form" onSubmit={handleSubmit} noValidate sx={{ mt: 1 }}>
<TextField
margin="normal"
required
fullWidth
id="email"
label="Email Address"
name="email"
autoComplete="email"
autoFocus
/>
<TextField
margin="normal"
required
fullWidth
name="password"
label="Password"
type="password"
id="password"
autoComplete="current-password"
/>
<FormControlLabel
control={<Checkbox value="remember" color="primary" />}
label="Remember me"
/>
<Button
type="submit"
fullWidth
variant="contained"
sx={{ mt: 3, mb: 2 }}
>
Sign In
</Button>
<Grid container>
<Grid item xs>
<Link href="#" variant="body2">
Forgot password?
</Link>
</Grid>
<Grid item>
<Link href="#" variant="body2">
{"Don't have an account? Sign Up"}
</Link>
</Grid>
</Grid>
</Box>
</Box>
</Container>
);
}
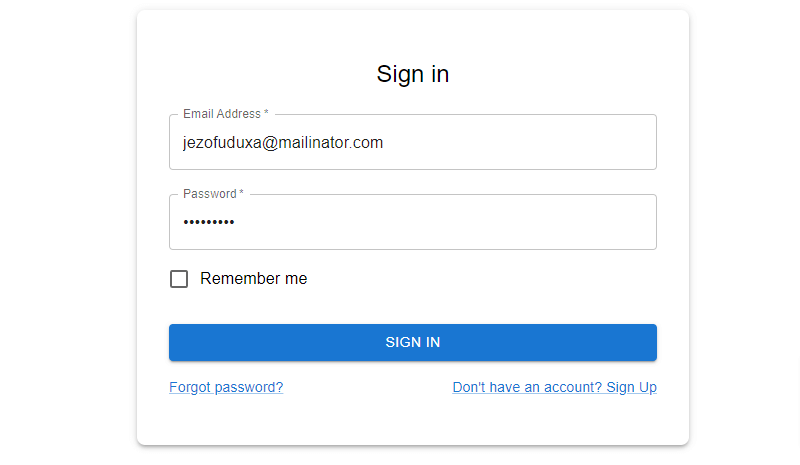
3. Building a React Material-UI 5 Login Page with a Side Image.
import Button from "@mui/material/Button";
import CssBaseline from "@mui/material/CssBaseline";
import TextField from "@mui/material/TextField";
import FormControlLabel from "@mui/material/FormControlLabel";
import Checkbox from "@mui/material/Checkbox";
import Link from "@mui/material/Link";
import Paper from "@mui/material/Paper";
import Box from "@mui/material/Box";
import Grid from "@mui/material/Grid";
import Typography from "@mui/material/Typography";
import { Container } from "@mui/material";
export default function SignInSide() {
const handleSubmit = (event) => {
event.preventDefault();
const data = new FormData(event.currentTarget);
console.log({
email: data.get("email"),
password: data.get("password"),
});
};
return (
<Container component="main" maxWidth="lg">
<Box
sx={{
marginTop: 8,
}}
>
<Grid container>
<CssBaseline />
<Grid
item
xs={false}
sm={4}
md={7}
sx={{
backgroundImage: "url(https://source.unsplash.com/random)",
backgroundRepeat: "no-repeat",
backgroundColor: (t) =>
t.palette.mode === "light"
? t.palette.grey[50]
: t.palette.grey[900],
backgroundSize: "cover",
backgroundPosition: "center",
}}
/>
<Grid
item
xs={12}
sm={8}
md={5}
component={Paper}
elevation={6}
square
>
<Box
sx={{
my: 8,
mx: 4,
display: "flex",
flexDirection: "column",
alignItems: "center",
}}
>
<Typography component="h1" variant="h5">
Sign in
</Typography>
<Box
component="form"
noValidate
onSubmit={handleSubmit}
sx={{ mt: 1 }}
>
<TextField
margin="normal"
required
fullWidth
id="email"
label="Email Address"
name="email"
autoComplete="email"
autoFocus
/>
<TextField
margin="normal"
required
fullWidth
name="password"
label="Password"
type="password"
id="password"
autoComplete="current-password"
/>
<FormControlLabel
control={<Checkbox value="remember" color="primary" />}
label="Remember me"
/>
<Button
type="submit"
fullWidth
variant="contained"
sx={{ mt: 3, mb: 2 }}
>
Sign In
</Button>
<Grid container>
<Grid item xs>
<Link href="#" variant="body2">
Forgot password?
</Link>
</Grid>
<Grid item>
<Link href="#" variant="body2">
{"Don't have an account? Sign Up"}
</Link>
</Grid>
</Grid>
</Box>
</Box>
</Grid>
</Grid>
</Box>
</Container>
);
}
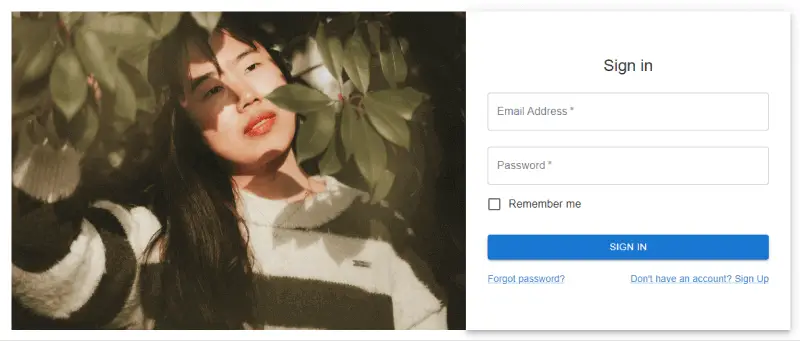
4. React mui 5 login form with validation using usestate hook.
import { useState } from 'react';
import TextField from '@mui/material/TextField';
import Button from '@mui/material/Button';
import FormControlLabel from '@mui/material/FormControlLabel';
import Checkbox from '@mui/material/Checkbox';
import Link from '@mui/material/Link';
import Box from '@mui/material/Box';
const LoginForm = () => {
const [formData, setFormData] = useState({
username: '',
password: '',
rememberMe: false,
});
const [errors, setErrors] = useState({
username: '',
password: '',
});
const validateForm = () => {
let valid = true;
const newErrors = { username: '', password: '' };
if (!formData.username) {
newErrors.username = 'Username is required';
valid = false;
}
// Password strength check
const passwordRegex = /^(?=.*[a-z])(?=.*[A-Z]).{6,}$/;
if (!formData.password || !passwordRegex.test(formData.password)) {
newErrors.password = 'Password must be at least 6 characters with at least one uppercase and one lowercase letter';
valid = false;
}
setErrors(newErrors);
return valid;
};
const handleSubmit = (e) => {
e.preventDefault();
if (validateForm()) {
// Add your login logic here
console.log('Login successful');
} else {
console.log('Login failed');
}
};
const handleChange = (e) => {
const { name, value, checked } = e.target;
setFormData({
...formData,
[name]: name === 'rememberMe' ? checked : value,
});
};
return (
<Box
component="form"
onSubmit={handleSubmit}
sx={{
maxWidth: '500px',
margin: 'auto',
padding: '20px',
borderRadius: '8px',
boxShadow: '0 4px 8px rgba(0,0,0,0.1)',
backgroundColor: 'white',
}}
>
<TextField
fullWidth
label="Username"
name="username"
value={formData.username}
onChange={handleChange}
error={Boolean(errors.username)}
helperText={errors.username}
margin="normal"
/>
<TextField
fullWidth
type="password"
label="Password"
name="password"
value={formData.password}
onChange={handleChange}
error={Boolean(errors.password)}
helperText={errors.password}
margin="normal"
sx={{ mt: 2 }}
/>
<FormControlLabel
control={<Checkbox checked={formData.rememberMe} onChange={handleChange} name="rememberMe" color="primary" />}
label="Remember Me"
sx={{ mt: 1, textAlign: 'left' }}
/>
<Button type="submit" variant="contained" color="primary" fullWidth sx={{ mt: 2 }}>
Login
</Button>
<Box sx={{ mt: 2, textAlign: 'center' }}>
<Link href="#" variant="body2">
Forgot Password?
</Link>
<Box mt={1}>
<Link href="#" variant="body2">
Don't have an account? Sign Up
</Link>
</Box>
</Box>
</Box>
);
};
export default LoginForm;
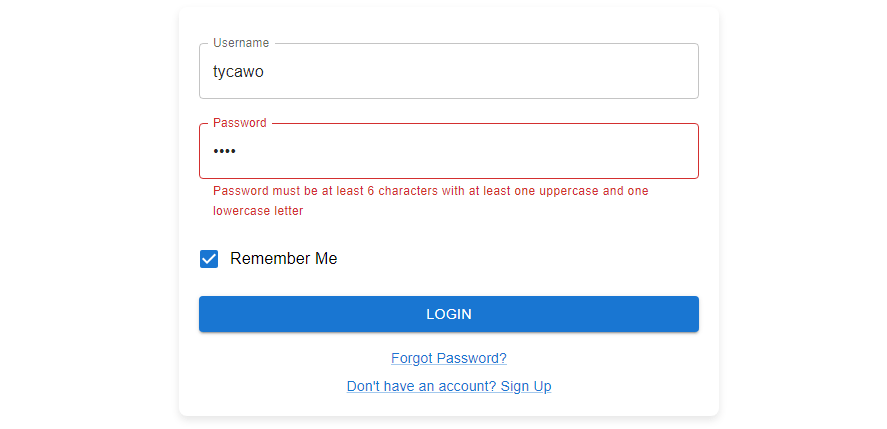
5. build a login form with validation in React Material UI 5 using react-hook-form.
How to Use react-hook-form in React with MUI 5
import { useForm } from 'react-hook-form';
import TextField from '@mui/material/TextField';
import Button from '@mui/material/Button';
import FormControlLabel from '@mui/material/FormControlLabel';
import Checkbox from '@mui/material/Checkbox';
import Link from '@mui/material/Link';
import Box from '@mui/material/Box';
import Typography from '@mui/material/Typography';
const LoginForm = () => {
const {
register,
handleSubmit,
formState: { errors },
} = useForm();
const onSubmit = (data) => {
// Add your login logic here using the data object
console.log('Login successful', data);
};
return (
<Box
component="form"
onSubmit={handleSubmit(onSubmit)}
sx={{
maxWidth: '500px',
margin: 'auto',
padding: '20px',
borderRadius: '8px',
boxShadow: '0 4px 8px rgba(0,0,0,0.1)',
backgroundColor: 'white',
}}
>
<Typography variant="h5" component="div" sx={{ mb: 2 }}>
Login Form
</Typography>
<TextField
fullWidth
label="Username"
{...register('username', {
required: 'Username is required',
minLength: {
value: 3,
message: 'Username must be at least 3 characters',
},
})}
error={Boolean(errors.username)}
helperText={errors.username?.message}
margin="normal"
/>
<TextField
fullWidth
type="password"
label="Password"
{...register('password', {
required: 'Password is required',
minLength: {
value: 6,
message: 'Password must be at least 6 characters',
},
})}
error={Boolean(errors.password)}
helperText={errors.password?.message}
margin="normal"
sx={{ mt: 2 }}
/>
<FormControlLabel
control={<Checkbox {...register('rememberMe')} color="primary" />}
label="Remember Me"
sx={{ mt: 1, textAlign: 'left' }}
/>
<Button type="submit" variant="contained" color="primary" fullWidth sx={{ mt: 2 }}>
Login
</Button>
<Box sx={{ mt: 2, textAlign: 'center' }}>
<Link href="#" variant="body2">
Forgot Password?
</Link>
<Box mt={1}>
<Link href="#" variant="body2">
Don't have an account? Sign Up
</Link>
</Box>
</Box>
</Box>
);
};
export default LoginForm;
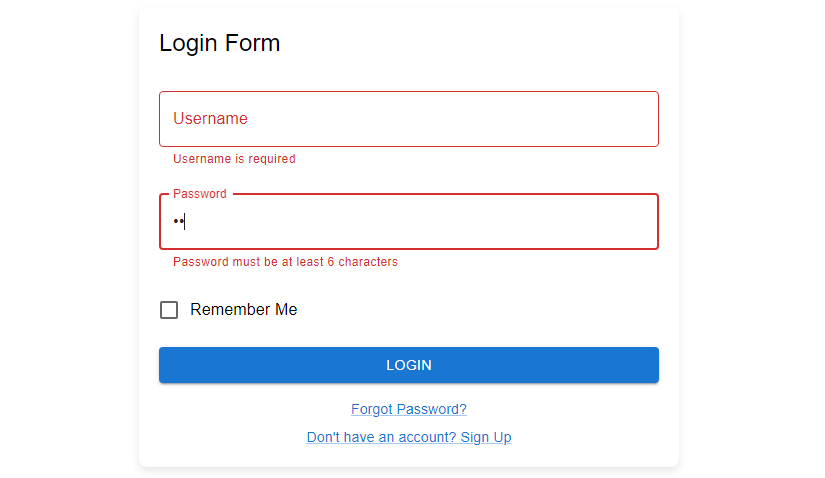