Building modern web applications has never been easier with the right tools. In this guide, we’ll walk you through the process of installing and setting up Supabase a powerful open-source backend-as-a-service platform with Tailwind CSS for sleek, responsive design, Vite for lightning-fast builds, and React for dynamic user interfaces. Whether you’re a seasoned developer or just getting started, this tutorial will help you integrate these technologies seamlessly, giving you a solid foundation for your next project. Let’s dive in and get your development environment up and running!
Prerequisites
Before we dive into the installation and setup process, make sure you have the following prerequisites in place:
- Node.js and npm: Ensure that you have Node.js and npm (Node Package Manager) installed on your system. You can download them from nodejs.org.
- A Supabase Account: Sign up for a free account at supabase.io if you haven’t already.
- Basic Understanding of React: Familiarity with React is beneficial, though not mandatory.
Setting Up Your Project
Start by creating a new React project using Vite. Open your terminal and run:
npm create vite@latest react-tailwind-supabase --template react
cd react-tailwind-supabase
Installing Tailwind CSS
Next, we’ll install Tailwind CSS and its dependencies. Run the following commands:
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
This will create two configuration files: tailwind.config.js
and postcss.config.js
.
Add the following lines to your tailwind.config.js
to set up the paths where Tailwind should look for class names:
/** @type {import('tailwindcss').Config} */
export default {
content: [
"./index.html",
"./src/**/*.{js,ts,jsx,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
Then, add the Tailwind directives to your src/index.css
file:
@tailwind base;
@tailwind components;
@tailwind utilities;
Installing Supabase
Now, let’s add Supabase to your project. Run:
npm install @supabase/supabase-js
Configuring Supabase
Create a new file in your src
directory named supabaseClient.js
and add the following code to initialize Supabase:
import { createClient } from '@supabase/supabase-js';
const supabaseUrl = 'YOUR_SUPABASE_URL';
const supabaseAnonKey = 'YOUR_SUPABASE_ANON_KEY';
export const supabase = createClient(supabaseUrl, supabaseAnonKey);
You can also create a .env
file to store your supabaseUrl
and supabaseAnonKey
values.
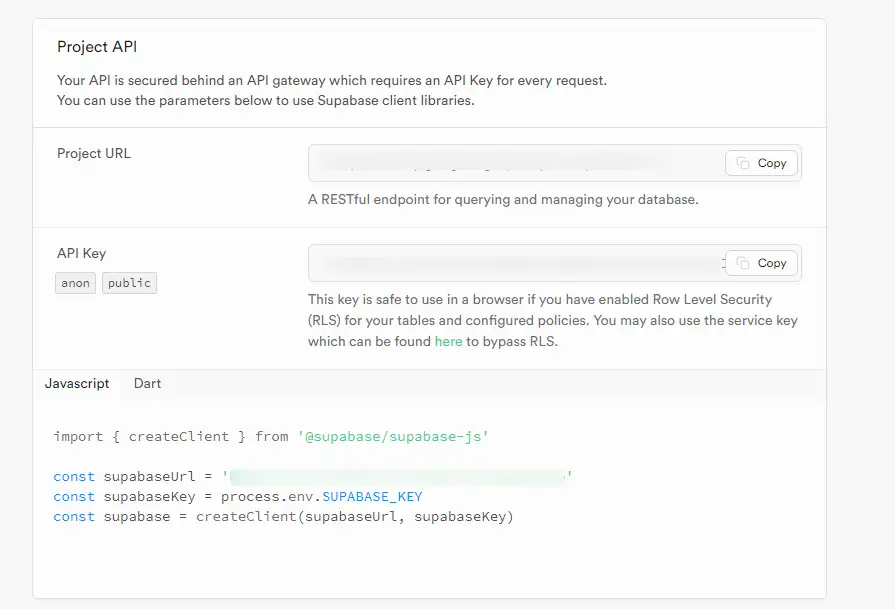
Now, go to the Supabase dashboard, navigate to the Database section, and create a new table.
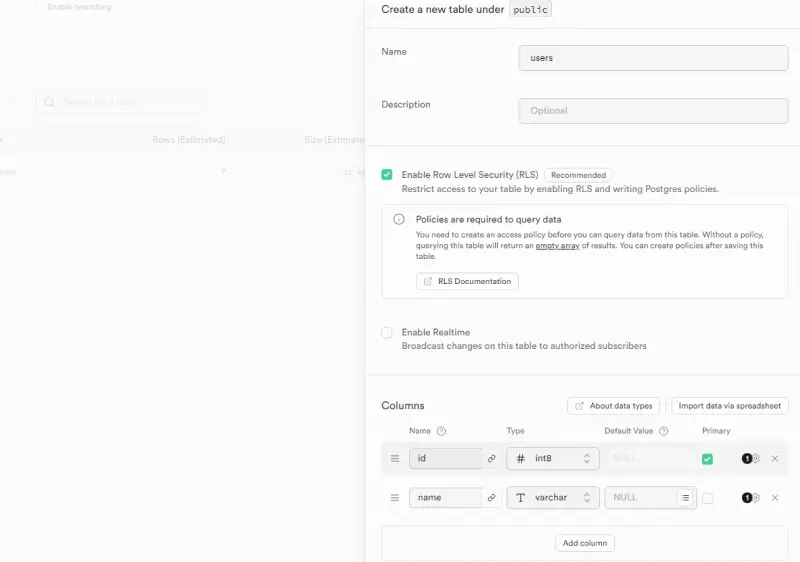
Add Supabase in Your React App
Now that Supabase is set up, you can start using it in your React App. let’s fetch and display data from a Supabase table.
import React, { useEffect, useState } from 'react';
import { supabase } from './supabaseClient';
const App = () => {
const [data, setData] = useState([]);
useEffect(() => {
const fetchData = async () => {
const { data: tableData, error } = await supabase
.from('users') // your table name
.select('*');
if (error) {
console.error('Error fetching data:', error);
} else {
setData(tableData);
}
};
fetchData();
}, []);
return (
<div className="container mx-auto p-4">
<h1 className="text-4xl font-bold mb-8">Welcome to frontendshape App</h1>
<div className="p-4">
<h1 className="text-2xl font-bold mb-4">Data from Supabase</h1>
<ul>
{data.map((item) => (
<li key={item.id} className="border-b py-2">
{item.name}
</li>
))}
</ul>
</div>
</div>
);
};
export default App;
If you are Using TypeScript.
import React, { useEffect, useState } from 'react';
import { supabase } from './supabaseClient';
// Define the type for your data items
interface User {
id: number;
name: string;
}
const App: React.FC = () => {
const [data, setData] = useState<User[]>([]);
useEffect(() => {
const fetchData = async () => {
const { data: tableData, error } = await supabase
.from<User>('users') // your table name
.select('*');
if (error) {
console.error('Error fetching data:', error);
} else {
setData(tableData || []);
}
};
fetchData();
}, []);
return (
<div className="container mx-auto p-4">
<h1 className="text-4xl font-bold mb-8">Welcome to frontendshape App</h1>
<div className="p-4">
<h1 className="text-2xl font-bold mb-4">Data from Supabase</h1>
<ul>
{data.map((item) => (
<li key={item.id} className="border-b py-2">
{item.name}
</li>
))}
</ul>
</div>
</div>
);
};
export default App;
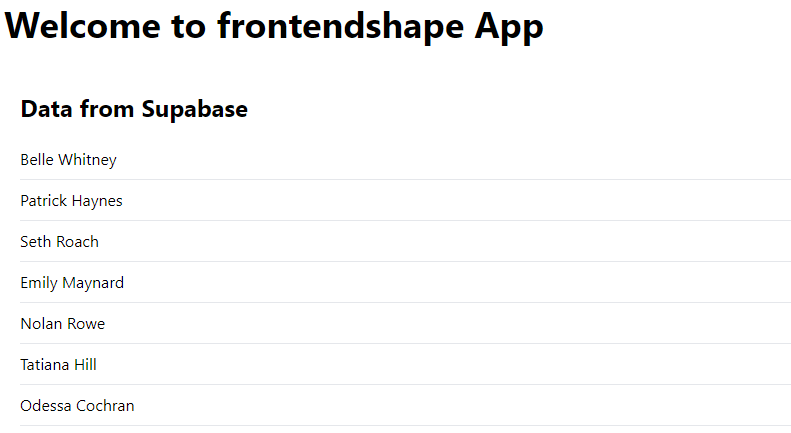
Running Your Project
With everything set up, you can now run your project to see it in action. In your terminal, execute:
npm run dev