In this tutorial, we’ll see how to use toastify in your React project using Tailwind CSS. Before you begin, ensure your project has React, and Tailwind CSS installed and configured.
Run below to install toastify in react app.
# with npm:
npm install --save react-toastify
# with yarn:
yarn add react-toastify
Make a basic toast feature in React using Tailwind CSS.
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
// minified version is also included
// import 'react-toastify/dist/ReactToastify.min.css';
export default function ToastDemo(){
const notify = () => toast("Wow so easy !");
return (
<div className='flex justify-center h-screen items-center'>
<button onClick={notify} className='px-2 py-2 bg-blue-500 text-white'>Notify !</button>
<ToastContainer />
</div>
);
}
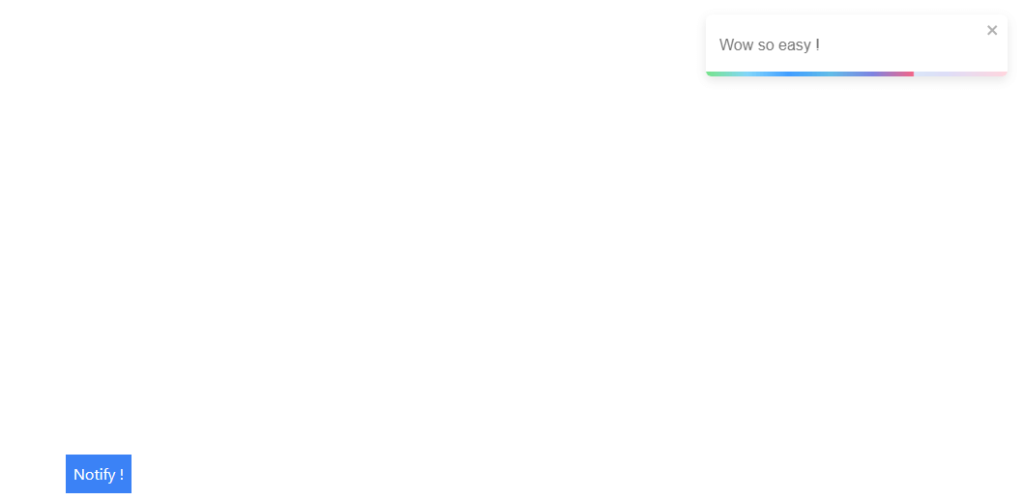
You can also change Toast notification position and color.
Toast Container
<ToastContainer
position="top-left"
autoClose={5000}
hideProgressBar={false}
newestOnTop={false}
closeOnClick
rtl={false}
pauseOnFocusLoss
draggable
pauseOnHover
theme="light"
transition: Bounce,
/>
Toast Emitter
toast.info('🦄 Wow so easy!', {
position: "top-left",
autoClose: 5000,
hideProgressBar: false,
closeOnClick: true,
pauseOnHover: true,
draggable: true,
progress: undefined,
theme: "light",
transition: Bounce,
});
Check more info about position https://fkhadra.github.io/react-toastify/introduction
See Also
How to Add Drag-and-Drop Image Upload with Dropzone in React Using Tailwind CSS