In this section, we’ll see Skeleton Loading in React.js with the help of Tailwind CSS. We’ll cover various aspects, including animating skeletons, displaying skeletons while fetching user data on page load, and creating a Skeleton Loading example in a React TypeScript application.
1. Create a Basic Skeleton Loading UI in React.js with Tailwind CSS.
export default function SkeletonLoader() {
return (
<div className="animate-pulse space-y-4 lg:max-w-xl w-full">
<div className="flex space-x-4">
<div className="rounded-full bg-gray-400 h-12 w-12"></div>
<div className="flex-1 space-y-4 py-1">
<div className="h-4 bg-gray-400 rounded w-3/4"></div>
<div className="space-y-2">
<div className="h-4 bg-gray-400 rounded"></div>
<div className="h-4 bg-gray-400 rounded w-5/6"></div>
</div>
</div>
</div>
</div>
);
}
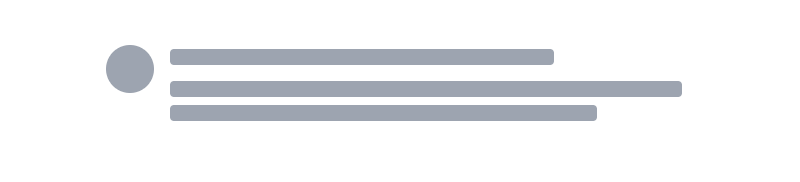
2. Building a on page Skeleton Loader in React.js with Tailwind CSS Using useState
and useEffect
Hooks.
import { useState, useEffect } from "react";
export default function App() {
const [profile, setProfile] = useState(null);
useEffect(() => {
const fetchData = () => {
setTimeout(() => {
return setProfile({
name: "Jane Doe",
bio: "Software Developer at Tech Corp.",
});
}, 3000);
};
fetchData();
}, []);
const SkeletonLoader = () => (
<div className="animate-pulse flex space-x-4 lg:max-w-xl w-full">
<div className="rounded-full bg-gray-400 h-12 w-12"></div>
<div className="flex-1 space-y-4 py-1">
<div className="h-4 bg-gray-400 rounded w-3/4"></div>
<div className="space-y-2">
<div className="h-4 bg-gray-400 rounded"></div>
<div className="h-4 bg-gray-400 rounded w-5/6"></div>
</div>
</div>
</div>
);
const UserProfile = ({ profile }) => (
<div className="flex space-x-4">
<div className="rounded-full bg-blue-500 h-12 w-12 flex items-center justify-center text-white font-bold">
JD
</div>
<div className="flex-1 space-y-4 py-1">
<div className="text-xl font-semibold">{profile.name}</div>
<div>{profile.bio}</div>
</div>
</div>
);
return (
<div className="App">
<header className="App-header p-5">
{!profile ? <SkeletonLoader /> : <UserProfile profile={profile} />}
</header>
</div>
);
}
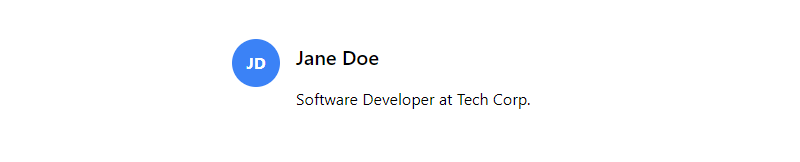
3. React Typescript with Tailwind CSS Skeleton Loader Using useState
and useEffect
Hooks.
import { useState, useEffect } from "react";
interface ProfileData {
name: string;
bio: string;
}
const App: React.FC = () => {
const [profile, setProfile] = useState<ProfileData | null>(null);
useEffect(() => {
const fetchData = () => {
setTimeout(() => {
setProfile({
name: "Jane Doe",
bio: "Software Developer at Tech Corp.",
});
}, 3000);
};
fetchData();
}, []);
const SkeletonLoader: React.FC = () => (
<div className="animate-pulse flex space-x-4 lg:max-w-xl w-full">
<div className="rounded-full bg-gray-400 h-12 w-12"></div>
<div className="flex-1 space-y-4 py-1">
<div className="h-4 bg-gray-400 rounded w-3/4"></div>
<div className="space-y-2">
<div className="h-4 bg-gray-400 rounded"></div>
<div className="h-4 bg-gray-400 rounded w-5/6"></div>
</div>
</div>
</div>
);
const UserProfile: React.FC<{ profile: ProfileData }> = ({ profile }) => (
<div className="flex space-x-4">
<div className="rounded-full bg-blue-500 h-12 w-12 flex items-center justify-center text-white font-bold">
JD
</div>
<div className="flex-1 space-y-4 py-1">
<div className="text-xl font-semibold">{profile.name}</div>
<div>{profile.bio}</div>
</div>
</div>
);
return (
<div className="App">
<header className="App-header p-5">
{!profile ? <SkeletonLoader /> : <UserProfile profile={profile} />}
</header>
</div>
);
};
export default App;
Sources
- react useState (react.dev)
- react useEffect (react.dev)
- Tailwind CSS (tailwindcss.com)
- typescriptlang.org
See Also
How to Add Drag-and-Drop Image Upload with Dropzone in React Using Tailwind CSS